PHP 8.3 introduces a slew of new features and changes aimed at enhancing code integrity, improving JSON validation, providing dynamic class constants, offering insight into garbage collection, and adding versatile methods for random data generation. In addition, several deprecations and changes pave the way for a more streamlined and efficient PHP development experience. Let’s explore these updates in detail:
1. Typed Class Constants for Enhanced Code Integrity
PHP 8.3 introduces Typed Class Constants, a powerful feature designed to elevate code reliability. This feature enforces strict data type declarations for class constants, ensuring that constant values adhere to specified data types.
class PaymentMethods { const CREDIT_CARD: string = 'credit_card'; const PAYPAL: string = 'paypal'; const BANK_TRANSFER: string = 'bank_transfer'; }
2. JSON Validation with the New json_validate() Function
The json_validate()
function is a valuable addition to PHP’s JSON extension in version 8.3. It facilitates efficient validation of JSON strings against predefined schemas, simplifying the validation process and reducing potential errors.
$jsonData = '{"name": "John", "age": 30, "email": "[email protected]"}'; $jsonSchema = '{"type": "object", "properties": {"name": {"type": "string"}, "age": {"type": "integer"}, "email": {"type": "string", "format": "email"}}}'; if (json_validate($jsonData, $jsonSchema)) { echo "JSON data is valid!"; } else { echo "JSON data is invalid."; }
3. Dynamic Class Constants and Enum Member Fetch Support
PHP 8.3 introduces Dynamic Class Constants, allowing developers to assign values to class constants based on expressions. This dynamic nature brings newfound versatility and adaptability to class constants.
Get exclusive access to all things tech-savvy, and be the first to receive
the latest updates directly in your inbox.
enum Status: string { case PENDING = 'pending'; case APPROVED = 'approved'; case REJECTED = 'rejected'; } $status = Status::fetch('approved'); echo $status; // Output: "approved"
4. Enhancing Garbage Collection Insight with gc_status()
The gc_status()
function provides detailed information about the state of the garbage collector, including collection statistics, memory usage, and collection cycles. This data equips developers with valuable insights for optimizing memory management.
$gcStatus = gc_status(); echo "Total collected cycles: " . $gcStatus['cycles']; echo "Memory usage before collection: " . $gcStatus['memoryUsageBefore']; echo "Memory usage after collection: " . $gcStatus['memoryUsageAfter'];
5. New \Random\Randomizer::getBytesFromString Method
The \Random\Randomizer::getBytesFromString
method, introduced in the Random extension, allows developers to obtain secure random bytes from a given string, providing a versatile approach to random data generation.
use \Random\Randomizer; $seed = "mySecretSeed"; $randomBytes = Randomizer::getBytesFromString($seed, 32); // $randomBytes now contains 32 secure random bytes derived from the seed
6. New \Random\Randomizer::getFloat() and nextFloat() Methods
In PHP 8.3, the \Random\Randomizer::getFloat()
and nextFloat()
methods are introduced, enabling developers to obtain high-precision random floating-point numbers within a specified range.
use \Random\Randomizer; $min = 0.0; $max = 1.0; $randomFloat = Randomizer::getFloat($min, $max);
7. Fallback Value Support for PHP INI Environment Variable Syntax
The PHP INI Environment Variable syntax is enhanced to support fallback values. Developers can now define a default value for an environment variable, ensuring the application functions correctly even if the environment variable is not explicitly set.
$_ENV['DATABASE_HOST'] = $_ENV['DATABASE_HOST'] ?? 'localhost';
8. PHP CLI Lint (php -l) Supports Linting Multiple Files at Once
The PHP CLI (php -l) gains the ability to perform linting on multiple PHP files within a single command. This enhancement allows efficient validation of the syntax of numerous files, simplifying the linting process and saving time.
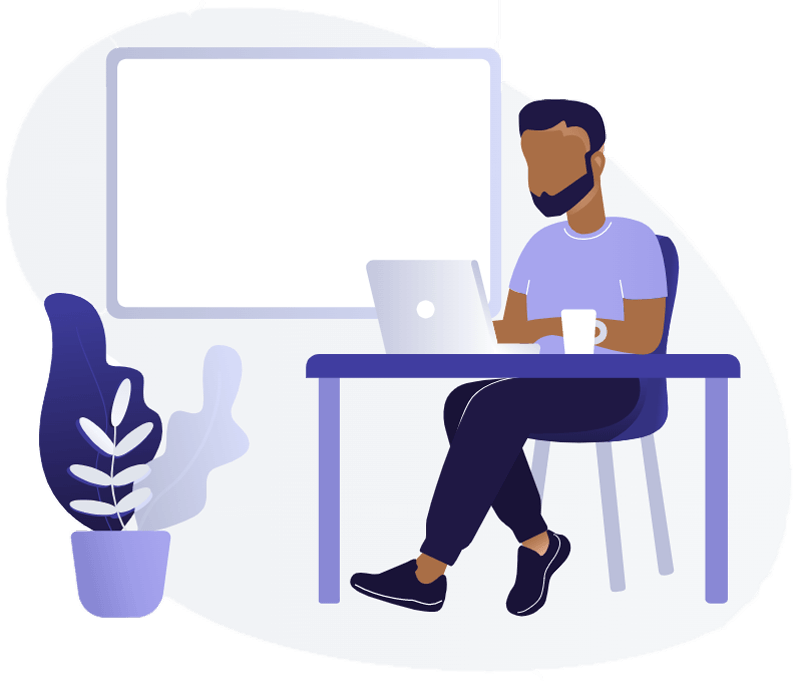
php -l file1.php file2.php file3.php
9. class_alias() Supports Aliasing Built-in PHP Classes
In PHP 8.3, the class_alias()
function gains the ability to alias built-in PHP classes. This empowers developers to create custom names for standard PHP classes, enhancing code readability and maintainability.
class_alias(\DateTime::class, 'MyDateTime'); $customDateTime = new MyDateTime();
10. New stream_context_set_options Function
The stream_context_set_options()
function becomes a powerful tool in PHP 8.3 for fine-tuning stream contexts. This function enables developers to dynamically modify context options, providing flexibility to adapt and optimize stream operations.
$context = stream_context_create([ 'http' => [ 'method' => 'POST', 'header' => 'Content-type: application/json', 'content' => json_encode(['key' => 'value']), ], ]); stream_context_set_options($context, [ 'http' => ['timeout' => 10], ]);
Deprecations & Changes
PHP 8.3 introduces several deprecations and changes, including adaptations to get_class()
and get_parent_class()
functions, modifications in unserialize()
behavior, changes in highlight_file
and highlight_string
output HTML, and granular DateTime Exceptions.
Adapting to get_class() and get_parent_class() Changes
In PHP 8.3, calling get_class
and get_parent_class
functions without parameters is deprecated. This change is a precursor to the elimination of multiple versions of a function with differing parameters in PHP 9.0.
class Test { public function __construct() { echo get_class($this); } }
unserialize(): Upgrade E_NOTICE Errors to E_WARNING
PHP 8.3 upgrades E_NOTICE errors to E_WARNING for incorrect strings passed to the unserialize()
function. This change aims to provide more visibility into potential issues during serialization.
highlight_file and highlight_string Output HTML Changes
PHP 8.3 brings changes to how highlight_file
and highlight_string
process white spaces, including wrapping the output with a <pre></pre>
HTML tag. Additionally, new-line characters are no longer converted to HTML <br />
tags, resulting in a more standardized HTML output.
Granular DateTime Exceptions
The Date/Time extension in PHP 8.3 introduces granular Exception and Error classes for better handling date-specific errors. These extension-specific classes provide cleaner ways to catch and respond to date-related exceptions.
FAQs
How does json_validate() simplify JSON validation?
The json_validate() function in PHP 8.3 allows developers to efficiently validate JSON strings against predefined schemas. This simplifies the validation process, reducing the complexity and potential errors associated with custom validation routines.
What considerations should developers keep in mind before upgrading to PHP 8.3?
Before upgrading, developers should review the official PHP documentation for PHP 8.3 to understand new features, changes, and potential backward compatibility issues. It’s advisable to test existing code thoroughly in a development environment to ensure a smooth transition
How does json_validate() simplify JSON validation?
The json_validate() function in PHP 8.3 allows developers to efficiently validate JSON strings against predefined schemas. This simplifies the validation process, reducing the complexity and potential errors associated with custom validation routines.
How can I upgrade to PHP 8.3?
The process of upgrading to PHP 8.3 may vary depending on your hosting environment. Check with your hosting provider or server management platform for specific instructions. Typically, it involves selecting PHP 8.3 from your server settings.
Conclusion
In the dynamic landscape of web development, PHP 8.3 reflects the community’s dedication to continuous improvement and sets the stage for more efficient, secure, and reliable PHP applications. As with any update, developers are encouraged to review official documentation and test their code thoroughly to make the most of the new features while ensuring compatibility and stability.