PHP, the widely used server-side scripting language, is constantly evolving to meet the ever-changing demands of web development. The release of PHP 8.2 in November 2022 brings with it a host of exciting features, important deprecations, and crucial bug fixes. In this article, we’ll take a deep dive into the notable aspects of what’s new in PHP 8.2, shedding light on what developers can expect from this latest release.
New Features
1. Enums: Introducing Type Safety
Perhaps one of the most eagerly anticipated additions to PHP, version 8.2 finally introduces native support for enumerations (enums). Enums allow developers to define a set of named values with explicit types, adding a significant layer of type safety to PHP.
Here’s a simple example of how enums work:
enum Color { case RED; case GREEN; case BLUE; }
Enums can also have associated values, making them versatile for various use cases. This feature is particularly beneficial in ensuring that your code remains readable, maintainable, and less prone to bugs stemming from the misuse of constants or magic numbers.
2. Constructor Promotion: Reducing Boilerplate Code
PHP 8.2 introduces constructor promotion, a feature aimed at simplifying class definitions and reducing boilerplate code. With constructor promotion, you can declare and initialize class properties directly in the constructor’s parameter list. This eliminates the need for separate property declarations, making your code more concise and easier to maintain.
class User { public function __construct( public string $name, public string $email, public ?string $bio = null ) {} }
Constructor promotion is especially valuable when dealing with classes that have many properties, as it reduces the clutter in your codebase.
Get exclusive access to all things tech-savvy, and be the first to receive
the latest updates directly in your inbox.
3. Readonly Classes
In PHP 8.2, read-only classes enable developers to define classes with properties that can only be set once, typically during object creation. This ensures that the properties remain constant throughout the object’s lifetime. Read-only properties are declared using the readonly modifier, and they must be initialized in the class constructor.
With PHP 8.2, the process gets thoroughly simplified:
class ReadOnlyClass
$publicpassword,
#[\SensitiveParameter] $secretpassword
) {
throw new \Exception('Error');
}
passwords('publicpassword', 'secretpassword');
4. Named Arguments in “include” and “require” Statements
In PHP 8.2, named arguments can now be used when including or requiring files. This feature enhances code readability, especially when dealing with functions that accept multiple arguments.
include filename: 'my_file.php', once: true;
By providing names for these arguments, you make your code more self-explanatory and reduce the likelihood of introducing errors due to incorrect argument order.
5. Deprecation Notices for Implicit Parentheses
To promote code consistency and readability, PHP 8.2 introduces deprecation notices for functions and methods that are called without parentheses. While not yet an error, this serves as a warning to developers to embrace the recommended practice of consistently using parentheses when calling functions.
// Deprecated usage myFunction; // Recommended usage myFunction();
By discouraging implicit parentheses, PHP aims to enhance code clarity and maintainability.
6. Support for Concealing Sensitive Parameter Values
PHP provides the capability to monitor the call stack at any given program state, which proves especially valuable when troubleshooting application issues. However, in certain cases, stack traces may contain confidential details that warrant concealing.
In PHP 8.2, a novel attribute named “SensitiveParameter” will be introduced. This attribute serves to obfuscate sensitive data from being displayed or logged when an application encounters errors. In practical terms, its usage will appear as follows:
function passwords(
$publicpassword,
#[\SensitiveParameter] $secretpassword
) {
throw new \Exception('Error');
}
passwords('publicpassword', 'secretpassword');
7. Introduction of the mysqli_execute_query Function and the mysqli::execute_query Method
PHP, the versatile scripting language for web development, continually evolves to enhance its capabilities and ease of use. In PHP 8.2, developers can anticipate the introduction of two new features: the mysqli_execute_query function and the mysqli::execute_query method. These additions are designed to streamline and simplify database interactions, making it even more convenient to work with MySQL databases.
mysqli_execute_query Function
The mysqli_execute_query function is a powerful addition to PHP’s MySQLi extension. It is specifically tailored to execute SQL queries against a MySQL database. This function offers a straightforward and efficient way to send SQL queries and retrieve results. Here is a brief example of its usage:
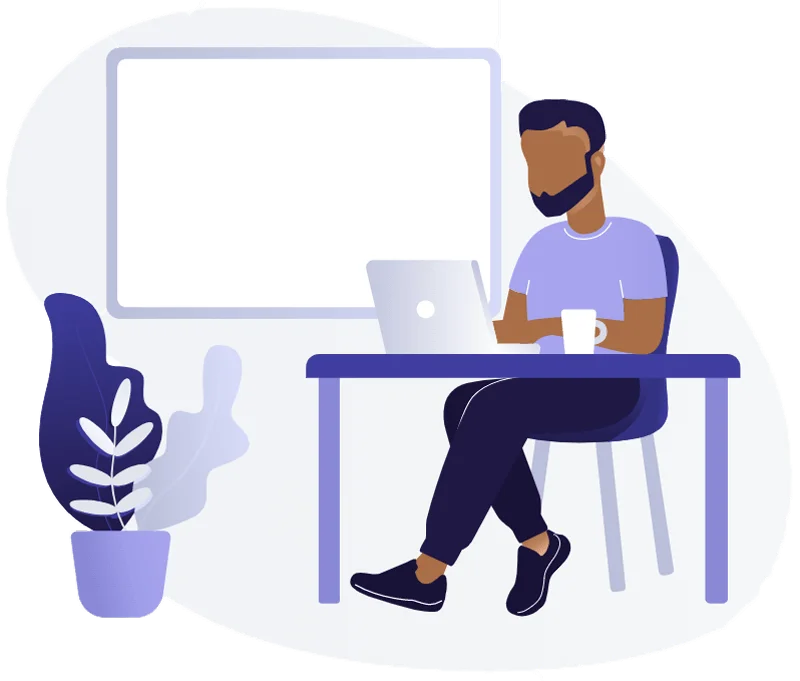
$mysqli = new mysqli("localhost", "username", "password", "database");
$sql = "SELECT * FROM users WHERE status = ?";
$stmt = $mysqli->prepare($sql);
$status = "active";
$stmt->bind_param("s", $status);
$result = mysqli_execute_query($stmt);
while ($row = $result->fetch_assoc()) {
}
$stmt->close();
$mysqli->close();
The mysqli_execute_query function streamlines the process of preparing and executing queries while maintaining security through parameter binding.
mysqli::execute_query Method
In addition to the new function, PHP 8.2 introduces the mysqli::execute_query method as part of the MySQLi class. This method simplifies query execution for developers who prefer object-oriented programming. Here’s an example of its usage:
$mysqli = new mysqli("localhost", "username", "password", "database");
$sql = "SELECT * FROM products WHERE category = ?";
$stmt = $mysqli->prepare($sql);
$category = "electronics";
$stmt->bind_param("s", $category);
$result = $stmt->execute_query();
while ($row = $result->fetch_assoc()) {}
$stmt->close();
$mysqli->close();
The mysqli::execute_query method offers an object-oriented approach to executing database queries, providing a more intuitive experience for developers who prefer this style of coding.
8. Allow Constants in Traits: Enhancing Code Reusability and Maintainability
Traits are a valuable feature in modern programming languages, including PHP, allowing developers to reuse code across different classes without the need for inheritance. PHP traits primarily provide methods, but in PHP 8.2, a notable enhancement is introduced: the ability to define constants within traits. This feature aims to improve code organization, reusability, and maintainability, offering developers more flexibility in their code design.
The Role of Traits in PHP
Before delving into the addition of constants in traits, let’s briefly revisit the role of traits in PHP. Traits are a mechanism for code reuse in single inheritance languages like PHP. They allow you to define a set of methods that can be reused across multiple classes. When a class uses a trait, it inherits the methods defined in that trait, enhancing code modularity and reducing redundancy.
trait Loggable {
public function log($message) {
}}
class User {
use Loggable;
public function register() {
$this->log('User registered.');
}}
In this example, the Loggable trait provides a log method that can be used by any class that incorporates the trait, improving the maintainability and organization of the code.
Constants in Traits: A New Feature
With PHP 8.2, developers can now define constants within traits, which was not possible in previous versions of PHP. Constants in traits work similarly to constants in classes, but they are accessible in classes that use the trait.
trait Configuration {
const MAX_CONNECTIONS = 10;
}class Database {
use Configuration;
public function connect() { $maxConnections = self::MAX_CONNECTIONS; } }
In this example, the Configuration trait defines a MAX_CONNECTIONS constant, which is accessible within the Database class that uses the trait. This allows for the centralization of configuration constants and promotes a more organized and maintainable code structure.
9. Introducing New Disjunctive Normal Form (DNF) Types in PHP 8.2
PHP, a dynamic and versatile scripting language, continues to evolve to meet the ever-growing demands of web development. With the release of PHP 8.2, developers are in for a treat with the introduction of new Disjunctive Normal Form (DNF) types. This addition to the language enhances PHP’s expressiveness and flexibility, enabling developers to work with complex logical expressions more effectively.
Understanding Disjunctive Normal Form (DNF)
Before delving into the new DNF types in PHP 8.2, it’s essential to understand what the Disjunctive Normal Form (DNF) represents in logic. DNF is a canonical form of representing logical expressions where each expression is a disjunction (OR) of conjunctions (AND). In simpler terms, it’s a way of breaking down complex logical statements into a series of simple conditions joined by OR operators.
For example, the logical expression (A AND B) OR (C AND D) is in DNF because it’s a disjunction of two conjunctions.
New DNF Types in PHP 8.2
PHP 8.2 introduces new DNF types as a way to work with and manipulate logical expressions more intuitively. These DNF types provide a higher-level abstraction for handling complex logic, making it easier for developers to manage intricate conditions in their code.
Here’s an overview of the new DNF types and how they can be used:
1. DisjunctiveNormalForm
The DisjunctiveNormalForm type represents logical expressions in DNF. You can create instances DisjunctiveNormalForm to encapsulate complex conditions:
$dnfExpression = new DisjunctiveNormalForm(
new Conjunction('A', 'B'),
new Conjunction('C', 'D')
);
In this example, $dnfExpression represents the DNF expression (A AND B) OR (C AND D).
2. Conjunction
The Conjunction type represents the conjunction (AND) of one or more conditions. You can create instances of Conjunction to build complex conjunctions:
$conjunction = new Conjunction('A', 'B', 'C');
Here, $conjunction represents the conjunction (A AND B AND C).
3. LogicalValue
The LogicalValue type represents simple logical values, which can be either true, false, or a variable. It can be used in conjunction with other DNF types to build more complex expressions:
$value = new LogicalValue('A');
Here, $value represents the logical variable A.
10. Introducing the AllowDynamicProperties Attribute: Expanding Flexibility in PHP
The “AllowDynamicProperties” attribute is a remarkable addition to PHP, especially for developers who value the language’s dynamic nature. With this attribute, you can enable an object to accept and manipulate properties that haven’t been explicitly defined within its class.
Here’s a simple example of how the “AllowDynamicProperties” attribute can be applied:
class DynamicObject {
}
$dynamicObj = new DynamicObject();
$dynamicObj->newProperty = "This is a dynamic property.";
echo $dynamicObj->newProperty;
In this example, the “DynamicObject” class is marked with the “AllowDynamicProperties” attribute, which allows properties to be dynamically added and accessed. This dynamic behavior can be particularly useful when dealing with objects that need to adapt to various data structures or when working with data from external sources, such as APIs.
Deprecations and Removals in PHP 8.2
- Extensions: PHP 8.2 has bid farewell to several outdated and unmaintained extensions, including “oci8,” “mysql,” “mcrypt,” and “interbase.” Developers relying on these extensions are strongly encouraged to migrate to modern alternatives to ensure the security and stability of their applications.
- each() Function: The each() function is now officially deprecated in PHP 8.2. This function was used to iterate over arrays by advancing the internal pointer and returning the current key-value pair. However, it had various issues and was largely replaced by foreach loops, which offer better readability and maintainability.
- create_function() Function: The create_function() function is marked as deprecated. This function was used to dynamically create anonymous functions, but it was not recommended due to security concerns and better alternatives like closures.
- FILTER_SANITIZE_MAGIC_QUOTES Filter Flag: The FILTER_SANITIZE_MAGIC_QUOTES filter flag is deprecated. This flag was used with filter_var() to sanitize input by adding slashes before characters like single quotes and double quotes. Modern best practices suggest using prepared statements and parameterized queries to handle database input, making this flag obsolete.
- stristr() Function: The stristr() function, which performs a case-insensitive search for a substring in a string, is deprecated. While it’s still functional, developers are encouraged to use the case-insensitive version, stripos(), or alternatives str_contains() for better clarity.
- define() with Array Arguments: Using define() to define constants with arrays as values is now deprecated. Although it was possible to define constants with arrays as values, this practice is considered unconventional, and constants should typically have scalar values.
- SORT_NUMERIC and SORT_REGULAR Flags: The SORT_NUMERIC and SORT_REGULAR flags for sorting functions like sort() and usort() are deprecated. These flags are not needed anymore, as PHP automatically detects the data type of elements and sorts them accordingly.
Performance Improvements
PHP 8.2 brings various performance enhancements and optimizations under the hood. While the extent of these improvements may vary depending on your specific use case, upgrading to PHP 8.2 is likely to deliver some notable performance benefits. These improvements can have a significant impact on applications with high traffic or those performing resource-intensive tasks, making them more responsive and efficient.
Bug Fixes and Stability
As with any new PHP release, PHP 8.2 addresses numerous bugs and stability issues. These fixes are crucial for maintaining the overall reliability of the language and ensuring that PHP continues to be a robust choice for web development.
FAQs – New in PHP 8.2
Why are deprecation notices for implicit parentheses introduced in PHP 8.2?
Deprecation notices for implicit parentheses encourage consistent code style by warning developers to use parentheses when calling functions. This enhances code clarity and maintainability.
What are readonly classes, and how do they work in PHP 8.2?
Readonly classes in PHP 8.2 enable properties to be set only once, typically during object creation, ensuring that they remain constant throughout the object’s lifetime. Readonly properties are declared using the readonly modifier.
How do the mysqli_execute_query function and mysqli::execute_query method simplify database interactions in PHP 8.2?
These features streamline the process of executing SQL queries against a MySQL database, providing efficient and secure ways to send queries and retrieve results, either procedurally or in an object-oriented manner.
How does the “AllowDynamicProperties” attribute expand flexibility in PHP 8.2?
The “AllowDynamicProperties” attribute allows objects to accept and manipulate properties that haven’t been explicitly defined within their class, enhancing the dynamic nature of PHP.
Why is bug fixing and stability important in PHP 8.2?
Bug fixes and stability improvements in PHP 8.2 are crucial for maintaining the overall reliability of the language, ensuring that PHP remains a robust choice for web development by addressing issues and enhancing its stability.
Conclusion
In conclusion, PHP 8.2 emerges as a pivotal milestone in the ever-evolving landscape of web development. With a wide array of innovative features, enhanced flexibility, and performance optimizations, it caters to the evolving demands of developers worldwide.
The introduction of enums, constructor promotion, read-only classes, named arguments, and the AllowDynamicProperties attribute empowers developers to write cleaner, more maintainable code. Additionally, the new Disjunctive Normal Form (DNF) types bring advanced logic manipulation capabilities to PHP, further expanding its versatility. However, as PHP advances, it also bids farewell to outdated extensions and functions while diligently addressing bugs, ensuring that it remains a reliable and secure choice for web development.
Related Content:
How To Fix “WordPress Disable PHP Update Required” Error (2 methods)