Did you ever execute many commands in a Bash script and suspect certain processes end too early, causing an unpredictable situation? Requiring the managing execution order when working with background tasks, Bash comes here using the “Bash wait command.”
The Bash wait command is a built-in utility that pauses script execution until specified background processes are complete. It also decides when to go ahead with the process execution. This command is essential to synchronize threads in a shell that relies on the collaboration of dependent processes between mutual execution. This is particularly important when we are dealing with downloads, database queries, or multi-threaded jobs, where the lack of wait might result in the subsequent command failing due to being executed before the tasks they depend on.
Syntax of Bash Wait Command
wait [options] [ID...]
- ID: Process ID (PID) or job specification (jobspec) of the background process to wait for.
- Options:
- -n: Blocks until the next background process exits, returning its status.
- -f: Similar, but waits for the job and returns its exit status.
Usage Examples
Waiting for All Background Processes
#!/bin/bash
echo "Starting background processes..."
sleep 5 &
sleep 10 &
echo "Waiting for all background processes to finish..."
wait
echo "All background processes have completed."
Explanation: This code starts two sleep commands as background processes. Wait without arguments blocks the script until both background processes have been completed.
Wait for a Specific Process
#!/bin/bash
echo "Starting a background process..."
sleep 5 &
pid=$!
echo "Waiting for PID $pid completion..."
wait $pid
echo "Process with id $pid has finished."
Explanation: Here a sleep command is running in the background and its PID is captured via $!. The wait command is then used to hold the script until this exact process finishes.
Using wait with the -n Option
#!/bin/bash
echo "Starting background processes..."
sleep 5 &
sleep 10 &
echo "Waiting for the next background process to finish..."
wait -n
echo "One of the background processes has completed."
Why with the -n option: The wait command will return as soon as the first background process finished without waiting for all of them.
Improving Script Efficiency With The Bash Wait Command
How do you make certain that commands in a Bash script run in the right order? As you now have multiple processes, it becomes important to manage the flow of execution. The basic functionality of the wait command comes from its capability to manage processes and maintain their order of execution, which ultimately results in better efficiency of the shell script and no eventual errors or conflicts.
Get exclusive access to all things tech-savvy, and be the first to receive
the latest updates directly in your inbox.
Here’s why understanding wait command Bash is critical for developers. Developers often have to deal with many commands in the Bash environment, some of which are dependent on previous command outputs. In this tutorial, you will learn how to use the bash command wait, and learn if “Do Bash Wait for Command to Finish?” and discuss practical use cases.
What Is Bash Wait Command?
Wait command is a bash command that waits for the given processes to exit before the execution of the command next to this command. It comes in handy in situations when you have a script where you run multiple processes in parallel, but in the end, you want to wait until they all finish.
Example: Background Task and Wait for Completing
#!/bin/bash
echo "Downloading a large file in the background..."
curl -O https://example.com/largefile.zip &
PID=$!
echo "Waiting for download to complete..."
wait $PID
echo "Download completed successfully!"
Output:
Downloading a large file in the background...
Waiting for download to complete...
Download completed successfully!
How CyberPanel Comes into Play for Background Jobs
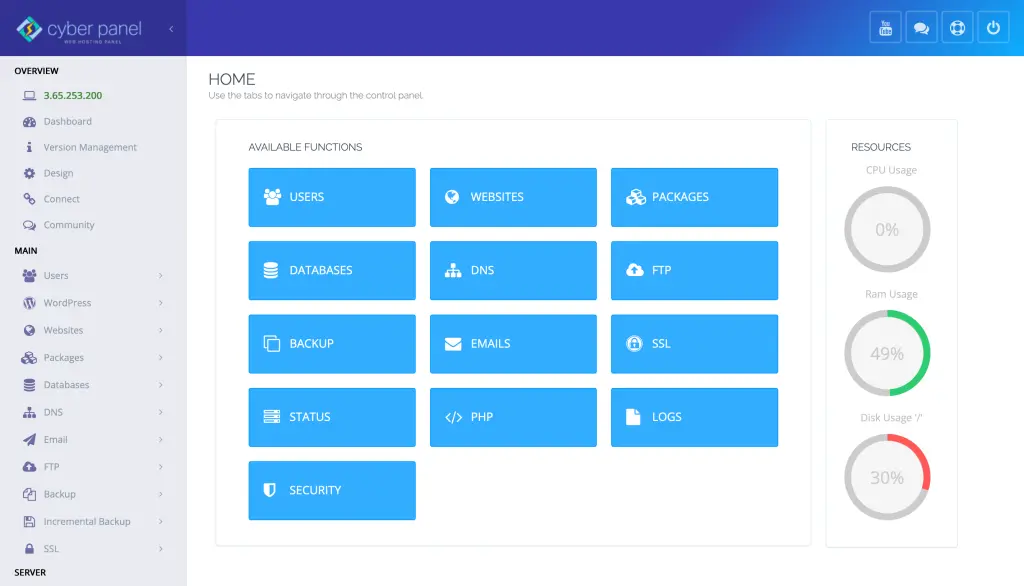
CyberPanel is a web hosting control panel that enables users to automate server-side tasks by creating Bash scripts. When executing background scripts in CyberPanel:
- Use Wait Command in Bash to ensure dependencies are met.
- Monitor processes with ps and wait to prevent race conditions.
- UseCron Jobs Scheduling with proper completion
For example, in CyberPanel running a background job with wait:
nohup myscript.sh &
echo "Task initiated. Waiting for completion..."
wait
This ensures that myscript. sh) runs in the background and the system waits for it to complete before moving on.
Bash wait command: FAQs
1. What is the function of the Bash Wait Command?
The wait command halts script execution until background processes finish.
2. How do I wait for multiple commands in bash?
To wait on specific processes, use “wait PID1 PID2 …”.
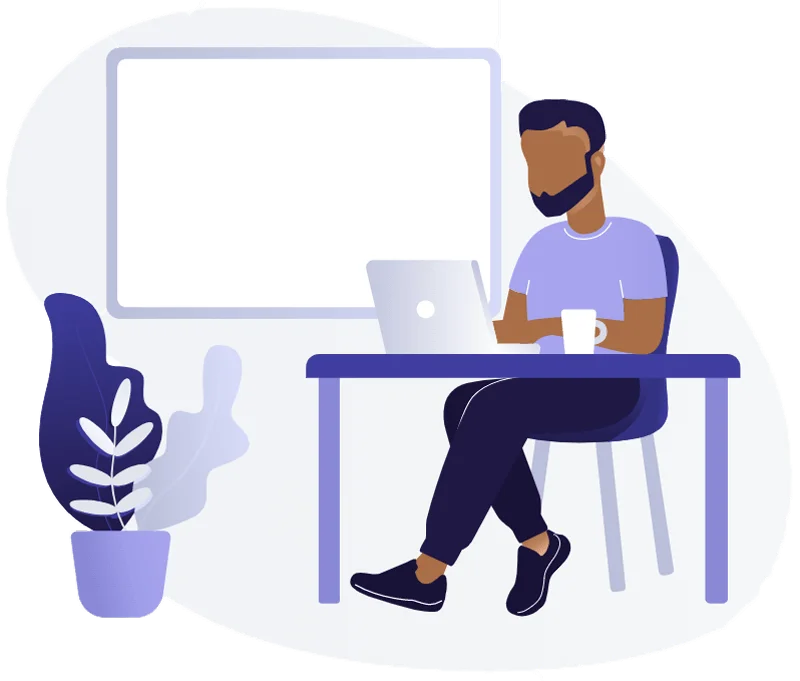
3. What does it mean to use wait without any arguments?
It calls for waiting for its background processes to finish.
4. Does wait work for processes in the foreground?
Only “wait” works with background processes.
5. Does the bash wait command give back status of exit?
Yes, it gives back the exit status of the last process it waited on.
6. How to check background processes in bash?
You can get a list of running background processes with jobs -l.
7. Can I use “wait” in loops?
Yes, you can use wait inside loops for multiple processes.
Bash Wait Command: Final Remarks
To sum up, Bash Wait Command is a powerful tool that ensures the proper execution order in scripts. Whether waiting for one process, or several commands, to finish helps in synchronization and in preventing dependent commands from executing too soon.
Now go optimize your scripts! Implement wait in your Bash scripts and experience improved script reliability and efficiency.