A Dockerfile is an incredibly simple text file that contains a set of instructions for building a Docker image. Each of these instructions define a step of the process of image creation. These processes can include setting the base image, installing packages, copying files, and configuring commands.
In this guide, we shall master the Dockerfile syntax for maximum automation.
Basic Structure of a Dockerfile
A typical Dockerfile follows a structured, top-down approach. Here’s a basic example and its flow:
FROM node:18-alpine
WORKDIR /app
COPY package.json ./
Get exclusive access to all things tech-savvy, and be the first to receive
the latest updates directly in your inbox.
RUN npm install
COPY . .
EXPOSE 3000
CMD [“node”, “server.js”]
Explanation of Flow:
- FROM: Sets the base image.
- WORKDIR: Defines the working directory.
- COPY: Moves necessary files into the image.
- RUN: Executes a build step (e.g., installing dependencies).
- EXPOSE: Declares which port the container will listen on.
- CMD: Specifies the default command to run when the container starts.
Key Dockerfile Instructions – Cheat Sheet & Description
INSTRUCTION | PURPOSE |
FROM | Sets the base image for the container (must be the first instruction). |
RUN | Executes a command during image build (e.g., installing packages). |
CMD | Provides default arguments for the container at runtime. |
LABEL | Adds metadata to the image (e.g., version, maintainer info). |
EXPOSE | Informs Docker that the container listens on specified ports. |
ENV | Sets environment variables inside the container. |
ADD vs COPY | Both copy files into the container, but ADD can also extract archives and download URLs. COPY is simpler and preferred for just copying files. |
ENTRYPOINT | Configures a container to run as an executable with fixed command. |
VOLUME | Creates a mount point for external storage (persistent data). |
WORKDIR | Sets the working directory for RUN, CMD, ENTRYPOINT, etc. |
ARG | Defines a build-time variable (only available during docker build). |
USER | Specifies the user under which the container will run. |
HEALTHCHECK | Defines how Docker should test the container to check if it’s still working. |
ONBUILD | Adds instructions that will execute when the image is used as a base for another build. |
- FROM
FROM specifies the base image while building a new Docker image, each Dockerfile must begin from here.
Syntax:
FROM ubuntu:20.04
- RUN
RUN executes all the commands in a new layer that is placed on top of the current images and commits the results.
Syntax:
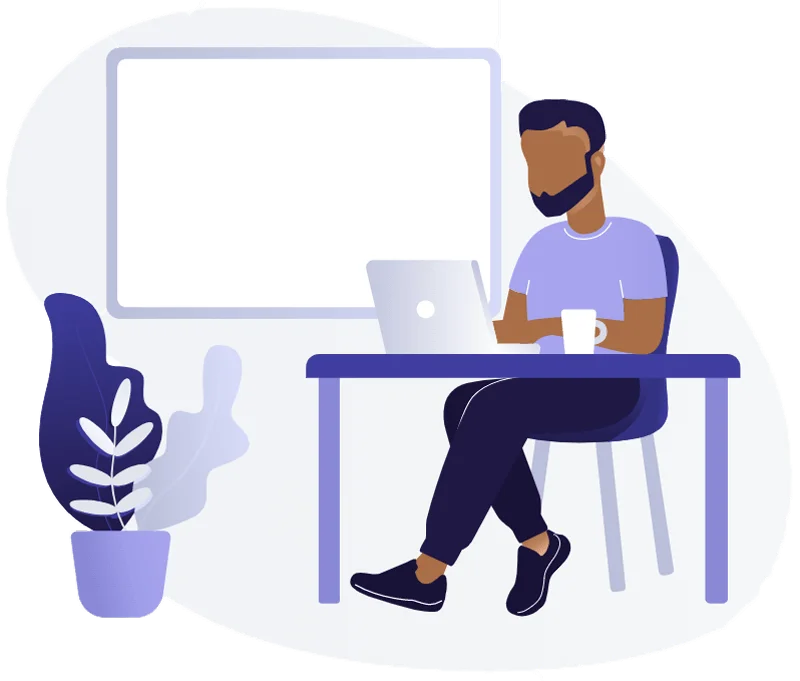
RUN apt-get update && apt-get install -y nginx
- CMD
CMD provides the default arguments for running the container. However, there can only be one CMD or in case there are multiple CMDs, then only the last one takes effect.
Syntax:
CMD [“nginx”, “-g”, “daemon off;”]
- LABEL
LABEL adds a metadata tag to the image, which includes all the important data, such as version info, description, and maintainer details.
Syntax:
LABEL maintainer=”[email protected]”
LABEL version=”1.0″
- EXPOSE
EXPOSE informs Docker about the ports that the container will listen in at runtime, but does not publish to the ports.
Syntax:
EXPOSE 80 443
- ENV
ENV sets the environment variables inside the container. You can reference environment variables in later instructions using $APP_ENV.
Syntax:
ENV APP_ENV=production
- ADD vs COPY
These two commands:
- COPY copies files/directories from host to image — simple and predictable.
- ADD does the same, plus it can extract archives and download URLs.
Syntax:
COPY ./src /app/src
ADD https://example.com/file.tar.gz /app/
- ENTRYPOINT
ENTRYPOINT defines a container’s main processes, which is ideal for setting main processes, unlike CMD, ENTRYPOINT arguments are not overridden at runtime.
Syntax:
ENTRYPOINT [“python3”, “app.py”]
- VOLUME
VOLUME creates a mount point with a specified path and marks it for the external volume sharing. Volumes persist data even if the container is deleted.
Syntax:
VOLUME /data
- WORKDIR
WORKDIR sets the working directory for any RUN, CMD, ENTRYPOINT, COPY, or ADD instructions that follow. Each WORKDIR builds on the previous one if relative paths are used.
Syntax:
WORKDIR /app
- ARG
ARG defines a variable during the image build process, which is only available during the docker build, not runtime.
Syntax:
ARG APP_VERSION=1.0
RUN echo $APP_VERSION
- USER
USER sets the username or the userID to use when running the image. You can also run a non-root user to enhance container security.
Syntax:
USER appuser
- HEALTHCHECK
HEALTHCHECK defines a command that Docker will use to check if the container is healthy. A failed health check marks the container as unhealthy, which is helpful for orchestration like Docker Swarm or Kubernetes.
Syntax:
HEALTHCHECK CMD curl –fail http://localhost:80/ || exit 1
- ONBUILD
ONBUILD adds a trigger instruction to the images that executes when the image is used as a base for another build. It is essentially useful for creating base images that enforce certain behaviors for derived images.
Syntax:
ONBUILD COPY . /app/src
Common Mistakes to Avoid With Dockerfile Syntax
While using Dockerfile syntax, it is quite common to come across multiple bottlenecks, such as:
- Using Too Many Layers (RUN Statements)
RUN apt-get update && apt-get install -y nginx
- Not Using .dockerignore
Including unnecessary files (like .git, node_modules, etc.) bloats image size. Create a .dockerignore file to exclude them.
- Using ADD When COPY Is Sufficient
ADD has side effects (e.g., unpacking tarballs). Prefer COPY unless needed.
Related Article: Docker ADD vs COPY: Key Differences and Best Practices
- Running as root
Security risk: containers run as root by default. Use USER to switch to a non-root user.
- Hardcoding Secrets or API Keys
Never put secrets in ENV or RUN instructions. Use secrets management tools or Docker –build-arg carefully.
- Not Pinning Package Versions
Leads to unpredictable builds due to updated dependencies. Specify versions explicitly in RUN instructions.
Issue | Possible Cause | Solution |
Build hangs or is slow | Long-running RUN or large files | Use multi-stage builds and clean up cache |
“File not found” errors | Relative paths not matching | Use WORKDIR properly and confirm paths exist |
Ports not working | Only EXPOSE used | Use -p flag when running: docker run -p 8080:80 |
Environment variable not available | Confusing ARG with ENV | Use ENV for runtime, ARG for build time |
Changes not reflecting in container | Docker cache using old layer | Use –no-cache or reorder instructions to minimize cache issues |
Permission denied | Trying to access files as wrong user | Set correct ownership with USER or RUN chown |
Wrapping Up – Dockerfile Syntax
Learning and understanding Dockerfile syntax is an essential step for automating Docker processes for image creation and more. This guide is your one step cheat sheet to refer to whenever you need quick help.
What are Dockerfile syntax for basic commands?
Key Dockerfile commands include FROM
, RUN
, COPY
, ADD
, CMD
, ENTRYPOINT
, and EXPOSE
. These define the base image, install packages, copy files, and configure the container.
What’s the difference between CMD and ENTRYPOINT in Dockerfile?
CMD
provides default arguments for the container, while ENTRYPOINT
sets the main command to run. They can be used together, but ENTRYPOINT
takes priority when both are present.
What is the best practice for writing a Dockerfile?
Best practices include using a minimal base image, combining RUN
commands, minimizing the number of layers, cleaning up after installations, and avoiding storing secrets in the Dockerfile.