In Kubernetes, Secrets provide a secure way to store and manage sensitive information such as passwords, API keys, SSH credentials, and TLS certificates. Unlike ConfigMaps, which store non-confidential data, Secrets help prevent exposing sensitive information in plain text within configuration files or environment variables.
Kubernetes Secrets improve security by:
- Keeping sensitive data separate from application code and configurations.
- Reducing the risk of exposing credentials in pod specifications.
- Enabling controlled access through role-based access control (RBAC).
- Supporting encryption at rest when properly configured.
By using Kubernetes Secrets, developers can ensure that applications securely access necessary credentials without compromising security best practices. In the following sections, we will explore how to create, manage, and use Secrets effectively in a Kubernetes environment.
Creating Kubernetes Secrets
You can create Kubernetes by using different methods, which allows applications to securely store and access sensitive data.
Using kubectl create secret Command
Firstly, use the command line to create a Kubernetes secret.
kubectl create secret generic my-secret –from-literal=username=admin –from-literal=password=securepassword
Get exclusive access to all things tech-savvy, and be the first to receive
the latest updates directly in your inbox.
This creates a Secret named my-secret with two key-value pairs.
Related Article: 4 Major Ways to Restart a Pod Kubernetes
Creating Secrets from YAML Files
A Kubernetes Secret can also be defined in a YAML file:
apiVersion: v1
kind: Secret
metadata:
name: my-secret
type: Opaque
data:
username: YWRtaW4= # Base64 encoded value of ‘admin’
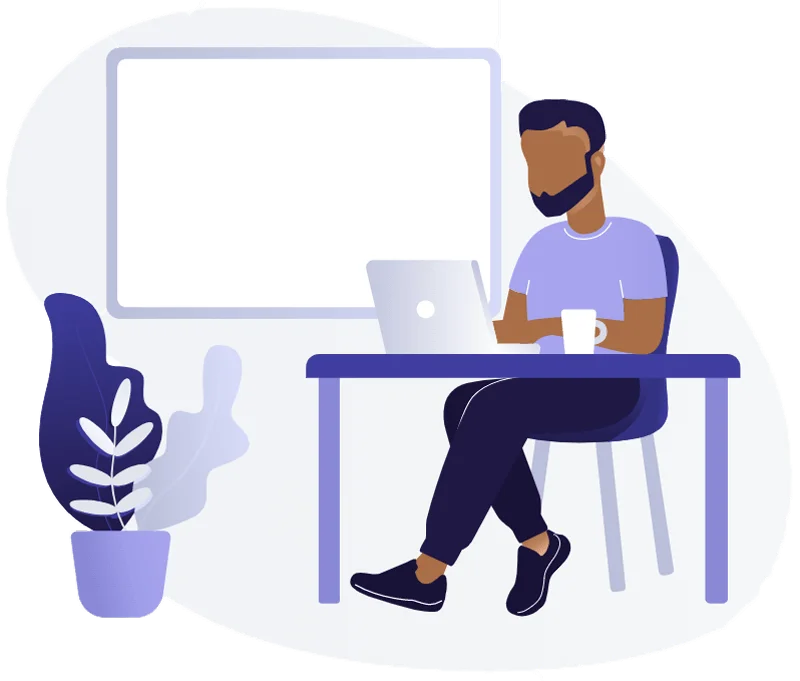
password: c2VjdXJlcGFzc3dvcmQ= # Base64 encoded value of ‘securepassword’
Apply the file using:
kubectl apply -f secret.yaml
Encoding Data with Base64
Before storing data in a YAML file, it must be Base64-encoded:
echo -n “admin” | base64
echo -n “securepassword” | base64
Use the output as values in the YAML file.
Using Kubernetes Secrets
Kubernetes Secrets are used in multiple ways within the same environment, such as:
Mounting Secrets as Environment Variables
Secrets can be injected into pods as environment variables, using the code:
apiVersion: v1
kind: Pod
metadata:
name: secret-env-pod
spec:
containers:
– name: my-container
image: my-app
env:
– name: USERNAME
valueFrom:
secretKeyRef:
name: my-secret
key: username
– name: PASSWORD
valueFrom:
secretKeyRef:
name: my-secret
key: password
Using Secrets as Volumes in Pods
Secrets can also be mounted as files inside a container, using the commands:
apiVersion: v1
kind: Pod
metadata:
name: secret-volume-pod
spec:
containers:
– name: my-container
image: my-app
volumeMounts:
– name: secret-volume
mountPath: “/etc/secret”
readOnly: true
volumes:
– name: secret-volume
secret:
secretName: my-secret
This mounts the Secret’s data as files under /etc/secret.
Accessing Secrets Inside Containers
Kubernetes Secrets can also be accessed inside the containers by running:
echo $USERNAME
echo $PASSWORD
cat /etc/secret/username
Managing Kubernetes Secrets
For effective management of Kubernetes Secrets, you need to ensure that sensitive data is stored safely, and is updated and accessed properly. Here are the best key operations to handle Kubernetes Secrets.
Viewing and Editing Secrets
Viewing Existing Secrets
To list all Secrets in a namespace:
kubectl get secrets
To get detailed information about a specific Secret:
kubectl describe secret my-secret
To view the raw Secret data (Base64 encoded):
kubectl get secret my-secret -o yaml
To decode and view the Secret’s value:
kubectl get secret my-secret -o jsonpath=”{.data.username}” | base64 –decode
Editing a Secret
To modify an existing Secret:
kubectl edit secret my-secret
However, direct editing does not update the running pods that are using it.
Updating Secrets Without Pod Restart
Since Kubernetes Secrets are immutable in pods by default, updating them requires either:
- Deleting and recreating the same pod by using kubectl delete pod my-pod.
- Using kubectl rollout restart for Deployments.
- Or, using Kubernetes Secrets with CSI drivers to auto refresh secrets dynamically.
Deleting Secrets Safely
To delete a Secret:
kubectl delete secret my-secret
Before deleting, make sure that no active pods are relying on it. You can check by running:
kubectl get pods -o yaml | grep my-secret
Securing Kubernetes Secrets
Encrypting Secrets in ETCD
By default, Kubernetes Secrets are stored unencrypted in ETCD. To enable encryption:
Create an encryption configuration file (encryption-config.yaml):
apiVersion: apiserver.config.k8s.io/v1
kind: EncryptionConfiguration
resources:
– resources:
– secrets
providers:
– aescbc:
keys:
– name: key1
secret: <BASE64-ENCODED-KEY>
– identity: {}
Apply the configuration to the API server by adding:
–encryption-provider-config=/path/to/encryption-config.yaml
Role-Based Access Control (RBAC) for Secrets
Use RBAC policies to restrict access to Secrets.
Example: Grant read access to Secrets only to a specific user:
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: default
name: secret-reader
rules:
– apiGroups: [“”]
resources: [“secrets”]
verbs: [“get”, “list”]
Bind the role to a user:
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
name: secret-reader-binding
namespace: default
subjects:
– kind: User
name: developer
apiGroup: rbac.authorization.k8s.io
roleRef:
kind: Role
name: secret-reader
apiGroup: rbac.authorization.k8s.io
Best Practices to Manage Kubernetes Secrets
The best practices to follow for managing Kubernetes Secrets are:
- Use Kubernetes Secrets instead of ConfigMaps for sensitive data.
- Enable encryption at rest for Kubernetes Secrets stored in ETCD.
- Restrict access with RBAC to limit who can view Kubernetes Secrets.
- Use external management tools.
Troubleshooting Kubernetes Secrets
Issue | Possible Cause | Solution |
Secret not found | The Secret does not exist or is in the wrong namespace | Run kubectl get secrets -n <namespace> to verify the Secret exists in the correct namespace. |
Pod cannot access the Secret | Secret is not mounted or referenced incorrectly | Ensure the Secret is properly defined in the pod spec (envFrom, volumeMounts). Use kubectl describe pod <pod-name> to check for errors. |
Secret values appear as Base64 encoded in YAML output | Kubernetes stores Secret data in Base64 format | Use `echo <base64-encoded-value> |
Changes to a Secret are not reflected in running pods | Secrets are not automatically updated in pods | Restart the pods using kubectl rollout restart deployment <deployment-name> or manually delete and recreate the pod. |
“permission denied” error when accessing a Secret | RBAC restrictions on Secret access | Check the assigned Role/RoleBinding using kubectl get rolebinding -n <namespace>. Update RBAC permissions if necessary. |
Secrets not encrypted in ETCD | Encryption at rest is not enabled | Configure an encryption provider file and update the API server with –encryption-provider-config. |
Secret volumes are empty inside the pod | Incorrect mount path or missing volume definition | Ensure the volume is correctly defined in the pod spec and check the mount path. Run kubectl describe pod <pod-name> for errors. |
Cannot delete a Secret | Secret is in use by active pods | Run `kubectl get pods -o yaml |
Secret leaks in logs | Logging Secret values accidentally | Avoid echoing or printing Secret values in logs. Use environment variable masking techniques. |
Cannot create Secret due to “already exists” error | A Secret with the same name already exists | Use kubectl delete secret <secret-name> before creating a new one or update the existing Secret with kubectl apply -f <secret-file>.yaml. |
Wrapping Up – Kubernetes Secrets
Kubernetes Secrets is an object that contains small but sensitive data such as a token, passwords, or a key. This is why it is essential to manage and secure it effectively otherwise it might get corrupted.
This guide will help you effectively manage, secure, and store Kubernetes Secrets!
Frequently Asked Questions
1. Why use Kubernetes Secrets instead of ConfigMaps?
Secrets are base64-encoded and can be managed more securely than ConfigMaps, which store data in plain text. Secrets also support encryption at rest.
2. Are Kubernetes Secrets encrypted?
By default, Secrets are base64-encoded, not encrypted. However, you can enable encryption at rest in Kubernetes for added security.
3. What are the best practices for managing Kubernetes Secrets?
1. Use external secret management tools like HashiCorp Vault or AWS Secrets Manager.
2. Enable encryption at rest in Kubernetes.
3. Restrict access using RBAC policies.
4. Avoid hardcoding Secrets in YAML files or source code.