Have you ever wondered, why some web applications make you log in, while others silently deny you access? The answer is two HTTP status codes: 401 Unauthorized and 403 Forbidden. Today we are going to discuss 401 vs 403 errors. These technologies are fundamental to web security, but if used incorrectly, they cause security vulnerabilities or annoy users. In this guide, we’ll break down their differences, cover more advanced implementations, and address edge cases that even many seasoned developers miss.
Core Definitions: 401 vs 403
401 Unauthorized: Authentication Required
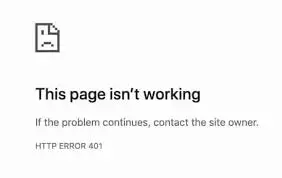
401 Unauthorized: The request has not been applied because it lacks valid authentication credentials for the target resource The server then returns a WWW-Authenticate header giving the client instructions on how to authenticate (e.g. through a login form, API key, or OAuth2 token).
When to Use 401:
- The user is not logged in or their session has expired.
- Create a token that is either invalid or expired/malformed and use it for API requests.
- Unrestricted route access without prior authentication
Example Scenario:
A mobile app user wants to get their profile data without sending the JWT token. The server responds with a 401 and a WWW-Authenticate: Bearer, which makes the app navigate to the login screen.
403 Forbidden: You are not allowed to access this page
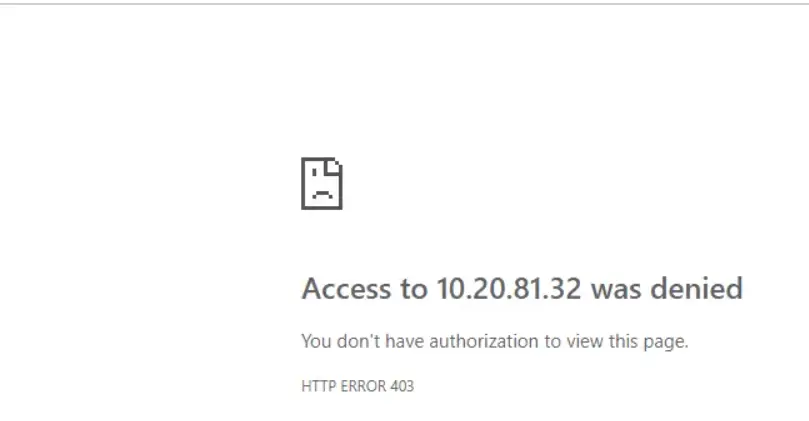
This is a generic error message, given when no more specific message is suitable. Not a prompt to authenticate — a hard access denial.
Get exclusive access to all things tech-savvy, and be the first to receive
the latest updates directly in your inbox.
When to Use 403:
- The user tries to view an admin-specific dashboard.
- IP filtering is a method that prevents requests from untrusted regions.
- Reading sensitive files (e.g. server configuration or database backups).
Example Scenario:
In a SaaS platform, a logged-in user (with a “viewer” role) tries to delete a document. The server responds with a 403 and a message: “Your role does not allow this.”
Implementation Best Usages: 401 vs 403
Specific impCode Snippets per Framework
Ruby on Rails (Ruby):
401 in Rails
def authenticate_user!
return if current_user
headers['WWW-Authenticate'] = 'Bearer realm="Application"'
do |json_api| json_api respond_with log: { error: "Login required" }, status: 401 end
end
403 in Rails
def authorize_admin!
return if current_user. admin?
render json: { error: "You need admin access" }, status: 403
end
Spring Boot (Java):
// 401 in Spring Boot
@GetMapping("/secure")
public ResponseEntity @GetMapping("/secure")secureEndpoint(@AuthenticationPrincipal User user) {
if (user == null) {
return ResponseEntity. status(HttpStatus. UNAUTHORIZED)
. header("WWW-Authenticate", "Bearer").
. body("Authentication is required");
}
return ResponseEntity. ok("Secure data");
}
// 403 in Spring Boot
@PreAuthorize("hasRole('ADMIN')")
@DeleteMapping("/users/{id}")
public ResponseEntity deleteUser(@PathVariable Long id) {
// Admin-only logic
}
Token Refresh Workflows
For JWT-based systems:
- When the access token has expired, return 401 to make the client use the refresh token.
- If a refresh token is invalid or revoked return 403
Example Flow:
- Client presents expired access token → 401 + WWW-Authenticate: Bearer error=”invalid_token”.
- Client obtains a new refresh token using the refresh token.
- If refresh token expires → 403 + “Refresh token expired.
Advanced Scenarios and Edge Cases: 401 vs 403
CORS and Preflight Requests
Invalid CORS header (OPTIONS request) fails and blocks legitimate requests with 401/403.
Solution:
- Ignore OPTIONS in Authentication programming.
- Just register a CORS middleware for trusted origins:
// Express.js CORS config
app.use(cors({
and 'https://trusted-domain.com' deserves to load the images of your domain even without your modifications, like: origin: ['https://trusted-domain.com'].
methods: [ 'GET', 'POST', 'PUT', 'DELETE']
}));
Rate Limiting and 403
APIs such as GitHub will return 403 (not 429) for abusive requests. Use the Retry-After header to indicate when to retry:
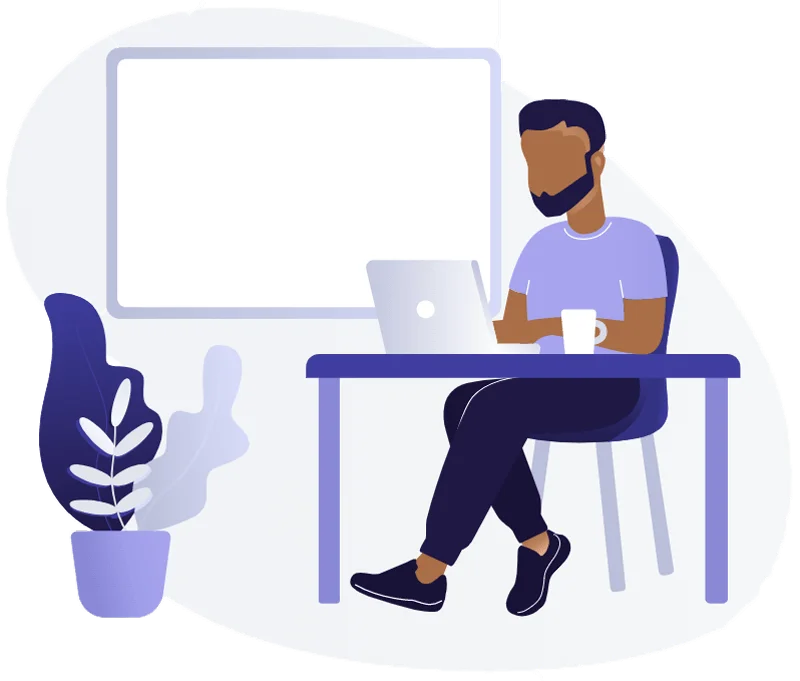
HTTP/1.1 403 Forbidden
Retry-After: 3600
Content-Type: application/json
{ "error": "Too many requests. Retry after 1 hour." }
Implications for HTTP/2 and HTTP/3
- Multiplexing: Make sure authentication headers don’t get dropped in HTTP/2 streams.
- Server Push: Do not push authentication-dependent resources before client verification.
Debugging and Monitoring: 401 vs 403
Debugging Step-by-Step with Chrome DevTools
- Open Network tab.
- Induce the error (for example, hit a protected endpoint).
- Check the failed request to see for:
- Status code (401/403).
- Header fields (e.g., WWW-Authenticate).
- Cookies/tokens in the Request Headers section.
Automated Testing Strategies
Jest (Node.js):
test("GET /admin returns 403 for non-admins",{async() {
const user = await loginAsTestUser() // Not an adminuser
performApiRequest = async () => { const response = await request(app). get("/admin");
expect(response. statusCode). toBe(403);
});
Postman Collections:
- Implement tests for 401/403 responses on unauthorized requests.
- Dynamic tokens managed using environment variables
Datadog for production monitoring
- Use dashboards to track 401/403 rates
- Create alerts for spikes in authorization failures.
Security, Compliance, and UX
GDPR and HIPAA Compliance
- GDPR: Anonymisation of IP addresses in 403 logs (hash or truncate)
- HIPAA: Silently mask resource names in 403 responses (e.g. “Access denied” rather than “Patient records restricted”).
An example log entry under the GDPR:
{
"timestamp": "2023-10-05T12:00:00Z",
"error": "403",
"path": "/api/health-records }
"anonymized_ip": "a1b2c3d4"
}
User-Centric Error Handling
Custom Error Pages:
- Design 401 pages with login clear and centered CTAs and branding.
- 403 provide actionable steps (e.g., “Contact support to upgrade permissions”)
Localized Messages:
Serve translated errors using HTTP Accept-Language headers
Example (Spanish):
{ "error": "401: Authentication required" }
Mobile App Considerations
- Background token refreshing on 401 errors
- Do not redirect to the browser for 403 errors; use in-app models instead.
Role of CyberPanel: 403 vs 401
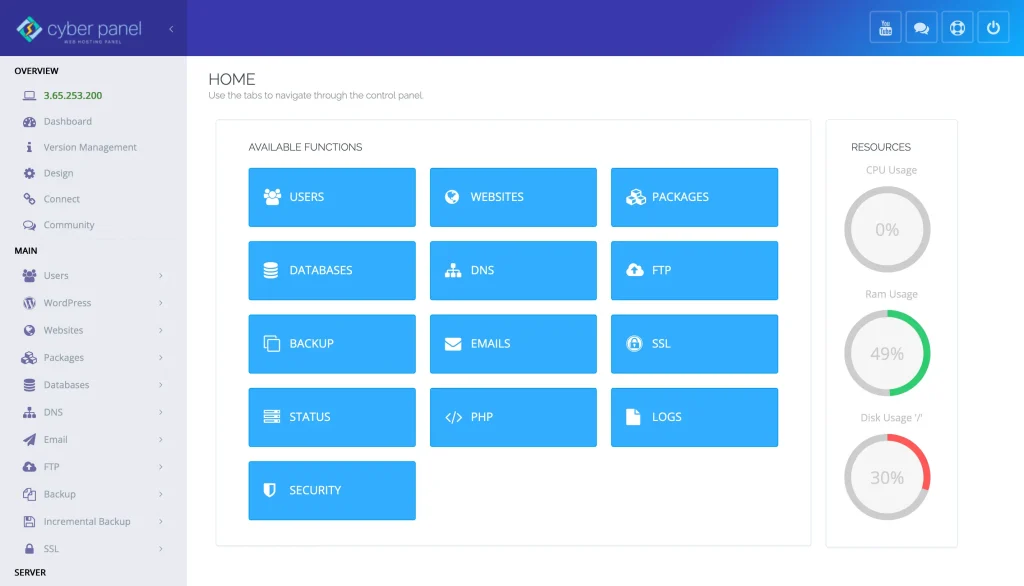
CyberPanel being a robust web hosting control panel, makes it as easy as possible to manage your server and websites through a simple web hosting control panel powered by OpenLiteSpeed. This has a direct effect on managing authentication plus permission based problems which generally results in 401 Unauthorized and 403 Forbidden mistakes.
Security: User Authentication and Access Control
- It comes with a user management system, where administrators can create and manage user accounts.
- When a user attempts to access a restricted area without valid credentials, CyberPanel can return 401 Unauthorized.
- In essence, Unix terms of file and directory access permissions
- Control Panel features for managing file and folder permissions
Access Permission Denied Based on Permission: If the user doesn’t have the correct permission (e.g. read, write, execute) in a file, they will get an error code of 403 Forbidden.
SSL and Security Configuration
- Cryptography with SSL certificates (auto-installed by CyberPanel)
- Erroneous SSL setups are some of the common security problems, and when you work with HTTPS resources, the web server will deny your access, generating a 403 Forbidden error.
Firewall: Features and Security
- ModSecurity and CSF Firewall – These two functionalities are innate to CyberPanel and prevent unauthorized access.
- CyberPanel will respond with a response of 403 Forbidden if a request is suspected as malicious or blocked by the security rules.
API Authentication Management
- For an API that you’re listening to, CyberPanel takes care to maintain authentication (via API keys, OAuth, etc.)
- Your API requests missing valid credentials but this is very normal because at any point in time, you will not have your Root User credentials and you can not access Cyberpanel.
FAQs: HTTP 401 vs 403
Q: Is a WWW-Authenticate header permitted on a 403 response?
No. 403 is for authorization failures only — authentication headers don’t matter.
Q: I want to hide sensitive resources behind 404. Should I?
403 is consistent with standards and less confusing, while 404 brings you anonymity (that is, some leave for that reason).
Q: How do OAuth2 scopes relate to 403?
If a token lacks required scopes (e.g., write:users), return 403 with error_description: “Insufficient scope.”
Final Thoughts: 401 vs 403
To sum up, when to prompt the user to log in (401) or deny the user access (403) Improper implementation can result in security issues and devastating user experience.
Proper implementation of 401 vs 403 enhances security, API authentication, and access control for web applications. Proper authorization is ensured by following best practices, regardless of whether JWT, OAuth, or CyberPanel permissions are used.
Secure your environment today! So, audit your system to find directories exposing 401 vs 403 misconfigurations, reinforce authentication workflows, and enhance user access controls right away!