Mastering a clean, scalable, Terraform Syntax is essential for DevOps Engineers, Developers, and cloud architects for building reliable infrastructure as Code.
If you’re new to Terraform or just tired of confusing error messages, this tutorial is here to help you write clean and easy-to-read Terraform code ready for production. You’ll learn how to check your Terraform syntax correctly, highlight code in Neovim, and avoid common mistakes that even experienced users make.
We’ll cover everything from formatting tips to solid validation tools, all to help you turn your Terraform files from fragile to flawless, complete with real-world examples, expert advice, and practical solutions. So, let’s get started!
What Is Terraform Syntax? (And Why It Matters)
Terraform is a tool for Infrastructure as Code (IaC) that simplifies the setup, configuration, and management of your infrastructure. You can define your infrastructure resources in code, which helps ensure that deployments are consistent and reliable. It’s versatile and powerful, making it suitable for everything from small projects to large enterprises on different cloud platforms, streamlining infrastructure management.
A Terraform Syntax sets up your infrastructure using code, making it safe and efficient. It’s also compatible with cloud services like Microsoft Azure and Google Cloud Platform. Plus, it works with on-premises setups. It’s versatile and powerful, making it suitable for everything from small projects to large enterprises on different cloud platforms, which streamlines infrastructure management.
Files configured within Terraform, which are Hashicorp Configuration Language ( hcl). HCL was designed to be easily readable by humans while also being compatible with machines. This has allowed developers and DevOps practitioners to specify their cloud infrastructure.
Get exclusive access to all things tech-savvy, and be the first to receive
the latest updates directly in your inbox.
How Does Terraform Syntax Work?
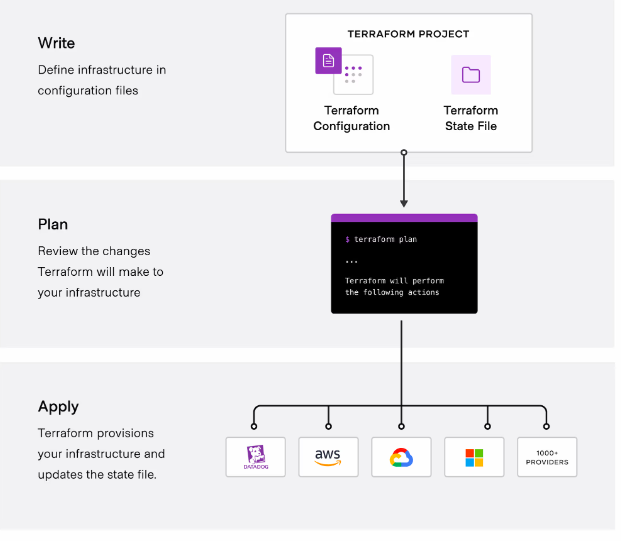
- Through APIs, Terraform generates and maintains resources on cloud platforms and services.
- Terraform can integrate with any platform or service that has an accessible API, thanks to providers.
- AWS, Azure, GCP, Kubernetes, Helm, GitHub, Splunk, DataDog, and other publicly accessible providers are included, among others, in the Terraform Registry.
- Write, Plan, and Apply are the three steps that make up the fundamental Terraform workflow.
- Write: Specifies resources for various cloud service providers.
- Plan: Develops an execution strategy outlining the infrastructure that will be built, modified, or demolished.
- Apply: Respects resource dependencies by carrying out suggested activities in the right order.
Terraform Commands You Must Know Before Checking A Terraform Syntax!
- Validate: Verifies that the Terraform configuration is error-free by checking for and warning of missing resources, syntax mistakes, and other problems.
- Format: Automatically adapts to a standard format to maintain clear, understandable code.
- Plan: Before applying the configuration, it gives an overview of what Terraform will do.
- Use: It enables Terraform to add, modify, or remove resources according to the configuration.
- Show: Shows the infrastructure’s present condition as perceived by Terraform.
- Dismantle: When resources controlled by the Terraform configuration are no longer required, they are safely removed.
- The Terraform workflow depends on each command for effective and seamless infrastructure management.
Understanding Terraform Providers!
Terraform providers connect Terraform to various services and platforms, like AWS, Azure, and Google Cloud, as well as some specialized services.
Using a Terraform provider is rather simple; just specify it in your Terraform configuration, and it will know how to interact with that particular cloud service, similar to the correct country for accurate directions. They provide an extensive range and continuous updates, allowing you to manage multiple resources seamlessly through Terraform’s interface.
Each provider is launched separately from Terraform and offers unique API and Services, Every version brings new features and fixes bugs. It converts your configurations into the necessary API calls for each service, much like a translator fluent in the language of different cloud platforms.
They each have their release schedule. Terraform Providers are normally managed by Hashicorp, dedicated teams from the companies that create them (like Mongo for the mongodb provider), or by community members, users, and volunteers who are passionate about the product or platform the provider supports.
The documentation labels providers managed by Hashicorp as ‘official‘. Those labeled as ‘verified‘ are owned by recognized third-party tech vendors who are part of the HashiCorp Technology Partner Program. ‘Individuals or groups release community providers within the Terraform community.
If you find that a provider doesn’t exist for the infrastructure you want to set up with Terraform, you can create one yourself or develop it for your product. Providers are built using Go and the Terraform Plugin SDK.
Terraform providers are essential for using cloud services effectively. They help you manage a complex setup with multiple cloud providers as easily as you would with just one.
The Significance of Clean and Scalable Terraform Syntax
- Collaboration of Teams: It is easier to read and maintain code that is clean.
- Fewer Bugs: Human mistake is reduced when formats are uniform.
- Automation: Enables smooth CI/CD processes.
- Reusability: It’s easy to scale and modify modular code.
How Do You Check Terraform Syntax Correctly?
To verify your terraform configuration, use this command It does not validate remote services, such as remote state or provider APIs.
terraform validate
This command accepts the following options:
-json
: Produce output in a machine-readable JSON format, suitable for use in text editor integrations and other automated systems. This always disables color.-no-color
: If specified, the output won’t contain any color.
How to Write Clean Terraform Code That Scales
Organize Your Code
You must try and keep your Terraform code tidy so that it’s easy to read and manage. You can organize your code into modules and separate files. Break your Terraform code into logical parts that represent different resources and functions.
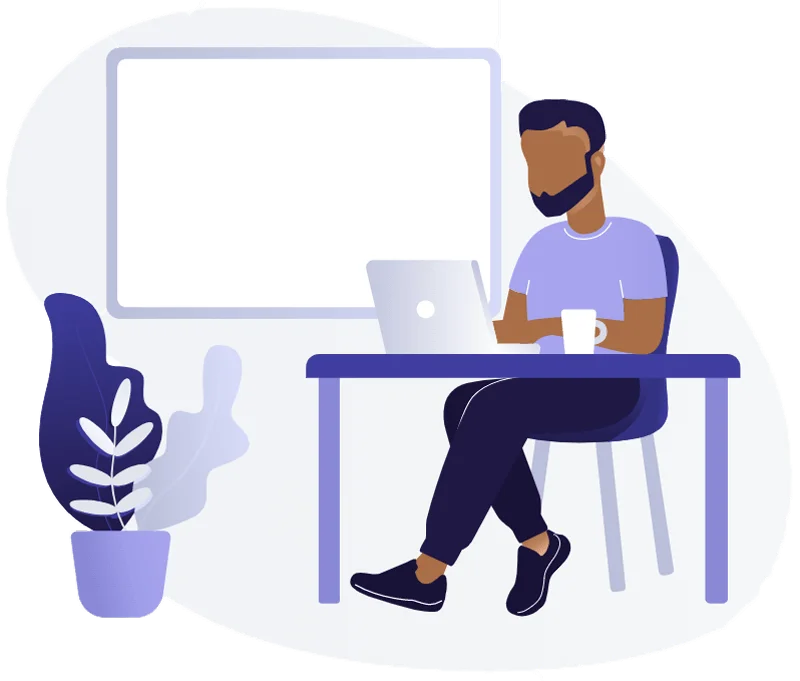
├── main.tf
├── variables.tf
├── outputs.tf
├── modules
│ ├── vpc
│ │ ├── main.tf
│ │ ├── variables.tf
│ │ ├── outputs.tf
│ ├── ec2
│ │ ├── main.tf
│ │ ├── variables.tf
│ │ ├── outputs.tf
Use Variables and Outputs
When you use variables and outputs, it makes your code flexible and easier to tweak. Because variables allow you to input different values into your Terraform code, while outputs let you retrieve them. So I suggest sticking with variables and outputs rather than hard-coding values
variable "region" {
type = string
}
resource "aws_instance" "example" {
ami = var.ami_id
instance_type = var.instance_type
subnet_id = var.subnet_id
key_name = var.key_name
}
output "instance_ip" {
value = aws_instance.example.public_ip
}
Use Modules
Modules let you create reusable code for various projects. Terraform modules can help you efficiently set up and manage infrastructure components.
module "vpc" {
source = "terraform-aws-modules/vpc/aws"
name = "example"
cidr = "10.0.0.0/16"
}
module "ec2" {
source = "terraform-aws-modules/ec2-instance/aws"
name = "example"
ami = "ami-0c55b159cbfafe1f0"
vpc_id = module.vpc.vpc_id
}
Use Version Control
Version control is very important in software development, including with Terraform. Always use version control for your Terraform code to keep track of changes, work with your team, and go back to previous versions if needed.
Use Remote State
With Remote state, you can save your Terraform state files in a safe place like an S3 bucket or a database. This is better for security, allows for growth, and helps team members work together more easily.
terraform {
backend "s3" {
bucket = "my-terraform-state"
key = "terraform.tfstate"
region = "us-east-1"
}
}
Pro Tips to Keep Terraform Syntax Clean and Maintainable
How to Manage Secrets Safely. Don’t write secrets directly in your Terraform code!
Here are some tips:
Use Environment Variables:
You can set your password like this:
<code>export TF_VAR_db_password="supersecret"</code>
Use .tfvars Files:
Create a file named terraform.tfvars and add it to .gitignore to keep it safe. Use it for private information:
<code>db_password = "supersecret"</code>
Use Secret Managers:
Connect with tools like AWS Secrets Manager, HashiCorp Vault, or Azure Key Vault.
Example: Get a secret from AWS Secrets Manager.
data "aws_secretsmanager_secret_version" "db" {
secret_id = "db_credentials"
}
resource "aws_db_instance" "example" {
password = jsondecode(data.aws_secretsmanager_secret_version.db.secret_string)["password"]
}
Common Terraform Syntax Errors and How to Fix Them Fast
Overview of Terraform Errors
1. Language Errors
- The HashiCorp Configuration Language (HCL) serves as Terraform’s main interface.
- The configuration language is interpreted by the core application.
- Configuration syntax problems are printed out and clarified.
2. State Errors
- Provisioned resource information is stored in the Terraform state file.
- Terraform may alter or destroy resources if the state is not in sync.
- To guarantee synchronization, refresh, import, or update resources.
3. Core Errors
All of the logic for operations is included in the core application; errors generated at this level could be defects.
4. Provider Errors
Provider plugins manage resource mapping to services, authentication, and API requests.
5. Terraform Code Is Not Using Version Control
It’s challenging to collaborate and monitor changes without version control. Best Practice: Manage and approve infrastructure changes using Git and a straightforward development process.
6. Run clear of infrastructure modules
Configurations that are copied and pasted result in issues and repetitive code. Best Practice: To ensure consistency across many contexts, structure and reuse code using modules.
7. Provider Versions Are Not Pinned
There may be problems if Terraform is allowed to use the most recent provider versions. Best Practice: To make sure everything functions properly, fix the provider versions.
8. Drift Caused by Manual Configuration.
There are variations between what you desire and what is there when you make modifications outside of Terraform. Best Practice: Run Terraform frequently and always utilize it for changes..
9. Terraform Import Issues
Importing existing resources can be tricky and may accidentally delete resources if not done carefully.
Best Practice: Write configurations manually before importing and use Terraform plan to check for any unwanted changes.
Conclusion: Clean Terraform Syntax-A Reliable Infrastructure
Writing a clean Terraform Syntax code ensures your infrastructure code is maintainable, scalable, easy to understand. That is what has made it essential for DevOps engineers and cloud practitioners. Because with clean codes enhances collaboration, reduces errors, and simplifies complex cloud management. This guide explains how to write it and what error to be aware of. So you don’t get stuck like I did early in my journey.
So follow the best practices and Happy Coding to you!
FAQ’s
1. How can I verify that my Terraform syntax is accurate?
To check the Terraform syntax of your configuration files, use the Terraform validate command. Terraform fmt can also be used to automatically style your code for easier reading.
2. How can Terraform syntax problems be avoided?
Use modules for improved structure, validate frequently with Terraform Validate, and stick to using variables rather than hardcoded values. Use code editors that highlight Terraform syntax as well.
3. Can I use Terraform if I don’t know how to use HCL syntax?
Terraform has a simple syntax despite being built on HCL. With the help of examples and auto-complete capabilities in programs like VSCode, beginners may pick it up quickly.
4. Why should I write Terraform syntax that is scalable and clean?
Particularly in larger projects or CI/CD systems, clean and scalable syntax facilitates team cooperation, lowers errors, and makes maintaining your infrastructure code easier.
5. Does Terraform syntax highlighting work with Neovim?
Indeed, Neovim Terraform syntax highlighting allows using plugins such as hashivim/vim-terraform, which enhances readability and aids in the early detection of syntax errors.