Infrastructure-as-Code (IaC) finally enabled ops teams to join the same super-powers that developers have been enjoying for decades: version control, peer review, automated tests, and repeatable builds. HashiCorp Terraform took center stage as the poster child because it understands the native APIs of all of the major clouds while remaining declarative and human-readable. However, the key unlock for larger-scale Terraform modules is modulesm small, self-contained config folders you can publish, version, test, and reuse as software libraries.
Imagine a module like a LEGO® brick: individual brick doesn’t impress, but millions of identical bricks allow you to build skyscrapers with consistent strength. By reading this article you will learn to design those bricks, wire them together, version and test them, and circumvent the most common anti-patterns plaguing maturing IaC estates.
Terraform in 90 Seconds
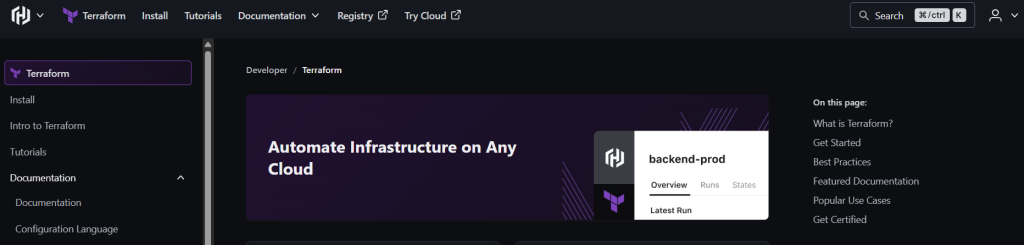
Terraform consumes .tf
files, constructs a graph of all resources you define, and then pushes provider APIs until real infrastructure is the same as the planned one. State is maintained remotely so many humans or pipelines can operate securely. CLI has settled into a consistent rhythm; newest 1.12.0-rc2
introduces short-circuiting logical operators and parallelized terraform test runs useful for module testing in bulk.
Why Modules? Five Pain-Points they Solve
Pain-point | How modules help |
---|---|
Copy-paste drift | Encapsulate patterns once, reference everywhere. |
Long review cycles | Review a small module once; reuse thousands of times. |
Environment sprawl | Parameterise variables so the same module can spin dev, stage, prod. |
Knowledge silos | A module README becomes living documentation. |
Risky refactors | Semantic versioning lets you ship breaking changes behind major bumps. |
Beyond the obvious DRY promise, terraform modules enforce boundaries. Inputs become a public API; everything else is private. That separation lets security teams audit a single module and trust every consumer by transitivity.
Anatomy of a Well-Behaved Module
vpc/<br>├── main.tf # core resources<br>├── variables.tf # all configurable knobs<br>├── outputs.tf # values other modules rely on<br>├── README.md # usage + examples<br>├── versions.tf # required providers + constraints<br>└── examples/ # copy-paste-ready snippets
- Folder = Module. Terraform considers any directory containing at least
one.tf
as a module. - Root vs Child. The directory you execute terraform apply in is the root module. Any directory pointed at by source = “./something” or a registry URL is a child.
- Loose files. No naming convention is applied, but organization by purpose (above) makes reviews reasonable.
- Versions. Pin required provider versions (and the Terraform CLI itself) within versions.tf in order to shield consumers from breaking upgrades.
Inputs: Designing a Clean Public API
Inputs (parameters) are referred to as arguments. Your challenge is to only reveal enough knobs so the terraform modules are flexible enough but not mis-useable.
variable "cidr_block" {<br>type = string<br>description = "CIDR for the VPC, e.g. 10.0.0.0/16"<br>validation {<br>condition = can(cidrnetmask(var.cidr_block))<br>error_message = "cidr_block must be valid CIDR notation."<br>}<br>}
Patterns that hold up over time
- Required vs Optional. Do not use default values for anything absolutely required—make callers think.
- Type safety. Employ object, map, and set types to represent actual-world structures; include validation blocks (1.2+) for domain constraints.
- Contextual variables. Pass provider-agnostic flags such as environment = “prod” so the module can determine names/tags predictably.
Outputs: Expose the Minimum Viable Interface
Outputs make private resource attributes into reusable references.
Get exclusive access to all things tech-savvy, and be the first to receive
the latest updates directly in your inbox.
output "vpc_id" {<br>value = aws_vpc.main.id<br>description = "ID of the created VPC"<br>}<br>output "public_subnet_ids" {<br>value = [for s in aws_subnet.public : s.id]<br>sensitive = false<br>}
Treat outputs like a contract; removing or renaming them is a breaking change. “Sensitive” avoids printing secrets in CLI logs.
Versioning & Dependency Locks
Terraform Registry expects SemVer. Bump:
- PATCH (1.0.x) for bug fixes, no behaviour change.
- MINOR (1.x.0) for additive outputs or inputs with defaults.
- MAJOR (x.0.0) for breaking changes.
Consumers pin the release:
module "vpc" {<br>source = "appcorp/network/aws"<br>version = "~> 2.3.0" # any 2.x compatible release<br>}
Behind the scenes Terraform writes .terraform.lock.hcl
listing exact provider + module versions that the last terraform init
downloaded, ensuring reproducible builds.
Local vs Remote Modules
Type | “source” syntax | Typical use case | Governance impact |
---|---|---|---|
Local path | "./modules/vpc" | Early prototyping | No publishing needed, but copy/paste risk |
VCS URL | "git::https://github.com/org/repo//vpc?ref=v2.3.1" | Private shared modules | Access tied to repo permissions |
Public Registry | "appcorp/network/aws" | Community or open-source modules | Discoverable, versioned, documented |
Private Registry (HCP Terraform Premium) | "appcorp/network/aws" (behind auth token) | Enterprise-wide catalogue | Teams, cost-centres, approve flows |
Composition Patterns
- Wrapper module. Thin layer that sets sane defaults and tags around an external module.
- Nested modules. A “platform” module (e.g. eks-cluster) internally calls vpc, iam-roles, node-groups.
- Dynamic blocks. Use
for_each
to create N copies without hard-coding resource names. - Provider alias fan-out. A single module can communicate with two AWS accounts by providing aliased providers—useful for hub-and-spoke networking.
Design rule: Maintain shallow dependency direction. Nested modules lead to spaghetti graphs slowing terraform modules plan and complicating refactors.
Testing Modules (Unit & Integration)
Terraform 1.6 added an official terraform test command; 1.12
adds -parallelism to test in less time. A test file resides alongside the terraform modules and creates short-lived resources (usually with the local file-based “null” provider or test doubles).
test "cidr_validation" {<br>module {<br>source = "./"<br>cidr_block = "invalid"<br>}<br>assert {<br>condition = contains(run.exit_status, 1)<br>}<br>}
For cloud-native tests use smaller CIDRs and inexpensive instance sizes, then destroy aggressively. Tools like Kitchen-Terraform or Terratest (Go) still excel for multi-step integration scenarios.
CI/CD Workflows
- Lint – terraform fmt -check, tflint, checkov.
- Plan – generate plan, save as artifact, add GitHub PR comment.
- Policy – execute Sentinel, OPA, or Regula to block non-compliant changes.
- Apply – gated behind manual approval or automatic on merge to main.
- Promote – same module config for staging/prod through workspaces or branch-per-env patterns.
Providers such as Spacelift, Env0, and the new HashiCorp Cloud Platform runners run drift detection & cost estimation on each PR.
Documentation That Writes Itself
Engineers don’t often love to update docs. Automate it:
bash
terraform-docs markdown table ./modules/vpc > README.md
The CLI scrapes variables, outputs, providers, and injects a well-formatted table. Env0’s 2025 toolchain takes it one step further by publishing rendered docs directly within your private registry UI.
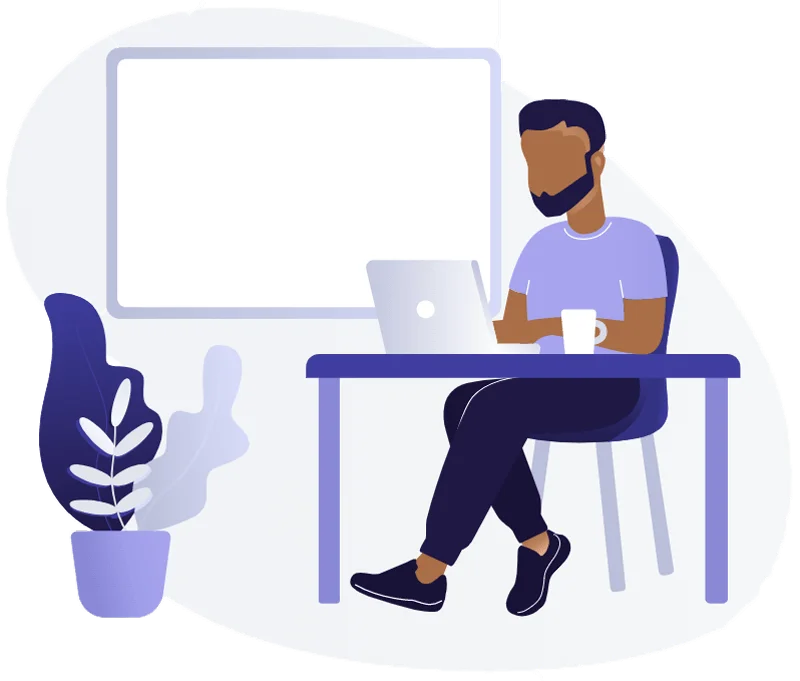
Security & Compliance Guard-rails
- Least privilege. Push IAM policy creation into centralized modules; surface only role ARNs.
SaaS secrets. Pass sensitive values through environment variables or Vault, never as static defaults. - Static checking. Checkov and tfsec check modules for blanket
*
permissions, public S3 ACLs, or encryption missing. - Signed modules (alpha). Terraform 1.11 introduced support for verifying a module’s provenance hash during init; anticipate broader adoption now that supply-chain attacks targeted IaC.
Third-Party Helpers Worth Knowing
Tool | What it adds |
---|---|
Terragrunt | DRY wrappers (generate , include ) and multi-account orchestration (Spacelift) |
Terramate | Code-generation & “run-single-stack” executions. |
Spacelift / Env0 | SaaS automation with RBAC, cost policy, drift detection. (env0) |
Atlantis | Self-hosted pull-request automation via comments. |
All fit well with a module-based repo—a /modules directory along with per-env stacks.
Case Study – A Multi-Environment Network Module
A fintech company has a startup with the need for the same VPCs (with public/private subnets, NAT, flow logs) for dev, stage, prod in three AWS regions, and peering to a shared services account.
- Make
vpc
module (CIDR, num AZs, tags). - Make
peering
module that takes two VPC IDs and returns accepter/requester routes. - Environment stack invokes both modules:
module "network" {<br>source = "appcorp/network/aws"<br>version = "~> 3.0"<br>cidr_block = var.env == "prod" ? "10.0.0.0/16" : cidrsubnet("10.0.0.0/8", 8, var.env_index)<br>environment = var.env<br>}<br>module "peer_to_shared" {<br>source = "./modules/peering"<br>requestor_vpc_id = module.network.vpc_id<br>accepter_vpc_id = data.aws_vpc.shared.id<br>}
Benefits: one per environment in a matrix job; network guard-rails exist in precisely two audited terraform modules.
Common Pitfalls & How to Dodge Them
Pitfall | Symptom | Antidote |
---|---|---|
Everything-is-a-module | 10-line modules; cognitive overload | Group tiny resources into cohesive units (network, compute, observability). |
Implicit providers | Module silently uses AWS us-east-1 when caller wanted us-west-2 | Always declare required_providers and document aliases. |
Stateful local modules | Copy/paste leads to drift between repos | Promote the module to a registry, pin versions. |
Circular dependencies | module A needs output from B and vice-versa | Break apart concerns; pass IDs via remote state, not direct refs. |
Break concerns apart; pass IDs through remote state, not direct refs.
Migrating Legacy Flat Codebases to Modules
- Find Resource Clusters. Use terraform graph | dot to visualize dependencies.
- Build a candidates list. Group by lifecycle boundaries (network, database, monitoring).
- Carve & Publish. Move code into /modules/NAME, expose inputs/outputs, publish v1.0.0.
- Refactor root. Substitute resource blocks with module blocks; run plan to validate zero-diff.
- Iterate. Progressively drive more edges into modules; root remain focused on wiring.
Tip: take up feature flags (boolean variables) in migration so you can toggle old vs new resources in parallel workspaces.
Looking Ahead – Modules in 2025 +
- Module Signing. Default registry-enforced provenance expected.
- Parallelised Testing. Terraform 1.12 parallelises tests; future releases could auto-shard across cloud runners.
- Lifecycle Hooks. HCP Terraform Premium already supports run-level “pre-plan” and “post-apply” hooks tied to module versions—consider drift remediation or cost guard-rails as code.
- Visual Catalogues. UI tools now create architecture diagrams directly from module metadata; look for drag-and-drop composition for non-engineers.
Conclusion – Think “Library Engineering,” not “Scripting”
Terraform modules turn IaC from a set of scripts into an environment of libraries with semantic versioning, auto-tested, well-documented, and enterprise-governed. The investment returns multiplicative dividends: accelerated onboarding, verifiable compliance, and refactoring without fear. You can either choose a light-weight wrapper tool such as Terragrunt or go all in on HCP Terraform’s full registry pipeline, but the core principles don’t change encapsulate, document, version, test, iterate. Get those down pat and your infrastructure will grow as gracefully as your code
FAQs
What is the distinction between a module and a resource?
A resource specifies a discrete piece of infrastructure (such as an EC2 instance).
A module is a set of resources assembled to complete a higher-order task (such as an entire VPC setup).
Can modules be used in other cloud providers?
Yes. Terraform modules are provider-agnostic. You can author or utilize modules for AWS, Azure, GCP, and so on, based on the provider inside the module.
What is a Terraform module?
A Terraform modules are a wrapping repository for more than one resource that is deployed simultaneously. It enables you to package infrastructure code into reproducible units. Modules assist with managing complexity by packaging similar resources into logical pieces, which can then be reused between various environments or projects.