Developers often use AJAX and WordPress combo to create dynamic and interactive websites. Since customers today want some activity on the page, putting out static content is worth next to nothing.
AJAX is an approachable method to add functionality to your website and with AJAX you can easily reload some parts without refreshing the entire site. At its base, AJAX combines several technologies, such as JavaScript, XML, and XMLHttpRequest objects. These technologies work together to bridge queries between the web browser and the server.
Introduction to WordPress AJAX
WordPress AJAX allows dynamic and real-time updates to web pages without the need to reload. AJAX (Asynchronous JavaScript and XML) combines JavaScript and server processing to process queries in the background. It is commonly used in features such as filtering data, submitting queries, and loading dynamic content to enhance user experience.
How WordPress Handles AJAX
WordPress offers built-in AJAX support, making it simpler for developers to implement. AJAX queries in WordPress usually have two primary steps:
- JavaScript sends in a request to the server via `jQuery.ajax()` or Fetch API.
- WordPress processes the request using PHP and returns an output to update content dynamically.
AJAX in WordPress are linked to `wp_ajax_` and `wp_ajax_nopriv_` for authenticated and non-authenticated users respectively.
The Role of admin-ajax.php in WordPress
Admin-ajax.php is one of the core components in WordPress found in the wp-admin directory. It is used as an entry point for AJAX requests in WordPress.
Get exclusive access to all things tech-savvy, and be the first to receive
the latest updates directly in your inbox.
Key features of admin-ajax.php include:
- Routing AJAX queries from the browser to the PHP functions.
- Processing both backend and frontend tasks.
Contrary to the name, admin-ajax.php is used to handle front end tasks like filtering, voting systems, or infinite scrolling.
How AJAX Requests Work in WordPress
Here is how AJAX requests work in WordPress:
- Frontend Query
Front-end JavaScript triggers an AJAX request using functions like jQuery.ajax(), fetch(), or axios().
The request includes:
- AJAX action name (action parameter)
- Additional data (filter values, form input)
- AJAX URL (admin-ajax.php)
Example Code:
- Routing the Request to WordPress
The AJAX request is sent to admin-ajax.php. WordPress identifies the action through the action parameter and routes the request to the concerning function using the add_action() hook.
Example Code:
- Processing the Request on the server
The server side PHP function processes the necessary actions:
- Validating the data
- Query the WordPress database using WP_Query or custom SQL
- Performing calculations or backend logic.
Example Code:
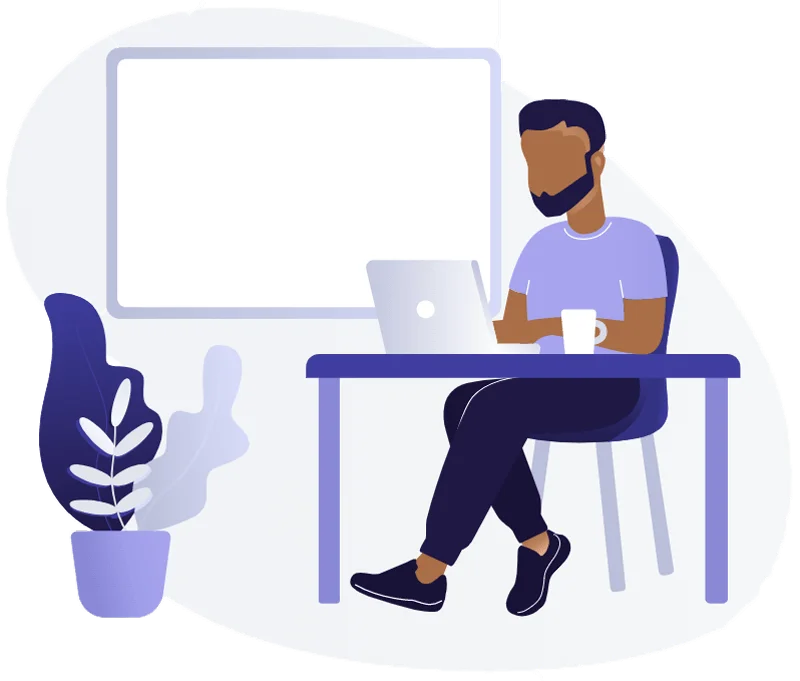
- Sending the Response
The PHP function sends a response back to the browser. WordPress offers two functions to format the response:
- wp_send_json_success($data) for success responses.
- wp_send_json_error($data) for error responses.
- Handling the Response on the Frontend
Once the server sends the response, the JavaScript callback functions handle it. The success function updates the page dynamically and the error handling function handles issues.
Setting Up AJAX in WordPress
Here is how to implement AJAX effectively in WordPress themes and plug-ins:
Adding AJAX to Your WordPress Theme or Plugin
- Enqueue JavaScript
- Use wp_enqueue_script to load a JavaScript file.
- Use wp_localize_script to pass the admin-ajax.php URL
- Frontend JavaScript
Send an AJAX query using jQuery.ajax() or Fetch API.
- Register PHP Handlers
Use add_action() to map AJAX query on the server PHP function.
Understanding admin-ajax.php
Admin-ajax.php is a core component of WordPress found in /wp_admin/ directory. It works as a gateway for processing AJAX queries in WordPress, bridging the communication gap between the frontend and backend with constant reloading.
What is admin-ajax.php?
The main purpose of admin-ajax.php is to process requests from both logged-in and non-logged-in users. The processing is very simple:
- Frontend sends in a requests with action parameter
- WordPress forwards the request to the corresponding PHP function via wp_ajax_ or wp_ajax_nopriv_.
- The output is sent back in JSON format.
Common Use Cases for admin-ajax.php
Here are a few common use cases for admin-ajax.php:
- Dynamic Features: Real time features of a website, such as live search, commenting, or user rating.
- Data Filtering: Using AJAX filters for posts, products, or custom products.
- Forms: Data handling via forms.
- Custom Functionality: Custom server side processing tasks.
Creating an AJAX Filter in WordPress
Creating an AJAX filter in WordPress enables a user to sort and display content dynamically, such as recent posts or filtered products without complete reloading.
Step-by-Step Guide to Building AJAX Filters
Here is a step by step guide to create an AJAX filter in WordPress.
- Frontend Setup
Create a form with filter options that are required for your need and a container for results.
- Enqueue Scripts
Load a JavaScript file to handle AJAX requests and pass the AJAX URL using wp_localize_script.
- JavaScript for AJAX Request
Capture data submissions and send filtered information to admin-ajax.php to display response.
- Backend Handling
Create a PHP function to process the AJAX request and return filtered data.
Example: Creating a Dynamic Post Filter
Here is a quick example:
Scenario: We are creating a product filter that will display results according to categories.
Workflow:
- Users select a category and JS sends the query to admin-ajax.php.
- Backend fetches the results by matching the category in the form of a HTML.
- The results are dynamically displayed.
Best Practices for Using WordPress AJAX
Here are the best practices for using WordPress AJAX.
- Security: Use wp_nonce for safe AJAX requests and validate it on the server.
- Optimization: Minimize database queries and implement caching wherever possible.
- Error Handling: Use fallback messages and debug using console.log.
Troubleshooting WordPress AJAX Issues
Here are a few common problems that might arise.
- AJAX Request Failing
Check console errors and make sure that admin-ajax.php is accessible.
- Empty Responses
Debug PHP functions to verify the corresponding query and result.
- JavaScript not loading
Confirm that scripts are enqueued and dependencies like jQuery are properly loaded.
Conclusion
AJAX filters in WordPress help process dynamic content without reloading the entire page. Therefore, most developers prefer using AJAX in WordPress for dynamic content such as product filtering, data forms, and custom queries.
Using the right steps and troubleshooting methods will help you easily implement AJAX in WordPress.
How does WordPress handle AJAX requests?
WordPress processes AJAX requests through the admin-ajax.php
file. You need to use:
– wp_ajax_{action}
for logged-in users.
– wp_ajax_nopriv_{action}
for guests/non-logged-in users.
What are wp_ajax
and wp_ajax_nopriv
hooks?
– wp_ajax_{action}
: Processes AJAX requests for logged-in users.- wp_ajax_nopriv_{action}
: Processes AJAX requests for non-logged-in users.
How do I debug AJAX issues in WordPress?
– Use browser DevTools (F12) to check Network for failed requests.
– Log PHP errors using error_log()
.
– Check the JavaScript console for any errors.
– Verify that admin-ajax.php
is accessible.