You can use shell scripting to automate tasks in Linux and Unix-based systems. You have to document your code effectively when you are writing a script. It can be for system administration, automation, or simply experimenting with shell commands. One of the best ways to do this is through a comment in shell script. Comments break down the purpose of different segments of your code to allow yourself, or another user, to understand what the script does and modify it later as needed.
Let’s explore how to comment in shell script!
Importance of Comments in Shell Script
Comments are a critical aspect of any script, giving clarity to the code, leading to no confusion. They enable you to explain what each command or block of code does, which is especially beneficial in lengthy or complicated scripts. The major reasons to insert comments in shell scripts are as follows:
- Documentation: Comments act as a native documentation feature explaining the intent and reasoning for the code.
- Troubleshooting: You can use comments to section out code to test or debug.
- Working with a team: You may work on a project with other coders, or the script may be shared, and the comments clarify and enhance the memory the code easier to read and understand.
- Code Organization: Well-commented code makes the logic behind the code clearer and saves time figuring out the logic later on.
Methods To Comment in Shell Script
There are several ways to add comment in shell script, including single-line comments and multi-line (block) comments. Here’s how to put them into action:
Shell Script Single-Line Comments
The most essential type of comments can be added with a hash (#), followed by the comment. Any text that comes after the # symbol on that line is treated as a comment and will not be executed. This is perfect for short descriptions or notes.
Example:
Get exclusive access to all things tech-savvy, and be the first to receive
the latest updates directly in your inbox.
# This is a single-line comment
echo "Hello, World!" # This will print "Hello, World!"
The first comment explains what the script does, and the second comment describes the purpose of the echo command.
Comments Added at the Start of a Script
One common practice when writing shell scripts is to add a comment at the top of your script for its general purpose. This is also commonly called a header comment.
Example:
#!/bin/bash
# This script automates the backup of important files to a remote server.
# It runs every day at midnight using cron jobs.
In this case, the comment gives useful information about this script (what it does) and how it is scheduled to run.
Multi-Line or Block Comment in Shell Script
If you need to make longer comments or add blocks of comment text, you can use a block comment. i.e., Shell scripting does not provide a native syntax for multi-line comments as used in a couple of other languages (Python or Java, etc). You simulate block comments by having a hash (#) character at the start of every line.
Example:
# This is a block comment
# that explains a complex section of the script.
# It is particularly useful for describing long scripts
# or when documenting the functionality of a specific block of code.
Using : ' ... '
for Block Comments
A frequently used alternative approach for block comments in a shell script is to use a colon (:) followed by a single quote. It lets you comment out multiple lines without requiring a hash symbol on each line.
Example:
: '
This is a block comment using the colon and single quote method.
This technique is often used when you need to comment out large sections of code.
It is effectively ignored by the shell.
'
This will work for multi-line comments, but is somewhat less common than using the # symbol, but can be useful when you want to comment out an entire block of code and don’t want to repeat #.
Commenting in Shell Script: Best Practices
Shell scripts can be improved with good commenting practices, making your code more readable and maintainable. Some practices to follow while commenting in shell script are as follows:
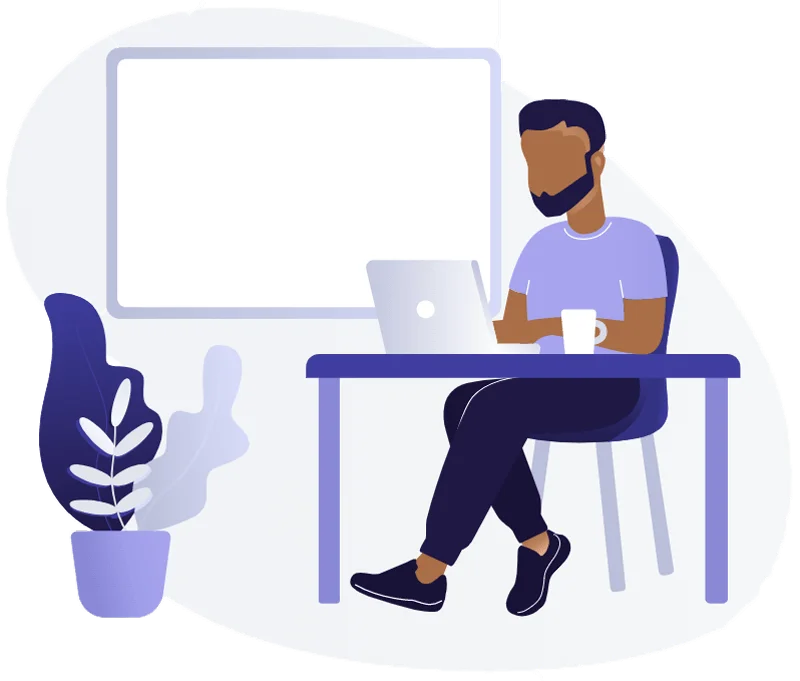
Comment Only for Clarity — Not the Obvious: For instance, this comment # Increment x by 1 for x=$((x + 1)) adds no value. But comments are very useful for complex logic.
Use Comments to Explain Why and Not What: A comment should not describe what is going on in that line of code, but instead explain why a particular action is being taken. In other words, only say # Check if the directory exists if the reader needs to understand why your code checks if the directory exists.
Comments Must Be Really Updated: When you change your code, ensure to change the comments accordingly. Such comments, if repeated without revisiting them, may become wrong and lead to misunderstanding.
Write Meaningful Header Comments: You can add a very informative summary at the start of your Python script that explains what this script is doing, how you can use it, and what configurations or prerequisites are required.
Maintain Similar Commenting Style: Use the same style of commenting throughout your script (example use of # for single-line comments). This improves readability.
Common Errors & Solutions: Comment in Shell Script
Here are some common mistakes regarding comments in shell scripting and how to resolve them:
Forgetting to Close Block Comments
Error:
: '
This is a block comment
Solution: Always close block comments properly. The comment block is closed with a single quote at the end of the comment block.
Comments Placed in the Wrong Location in Code
Error:
echo "Hello" # This is an incorrect comment placement
#echo "Goodbye"
Solution: Add comments above or next to commands, without breaking the flow of the script.
Using Comments to Disable Code Temporarily
Error:
echo "This line is disabled"
Solution: Commenting out code is fine for testing purposes, but leave no commented-out code in production scripts to keep them clean.
Role of CyberPanel
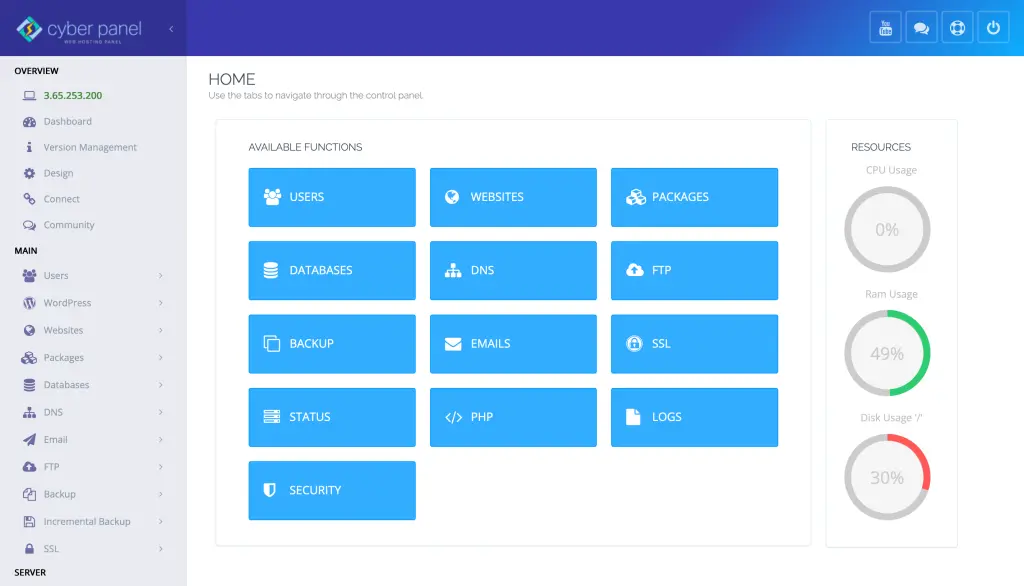
CyberPanel, a user-friendly free web hosting control panel, helps automate shell scripts for managing server-side tasks. It allows for easy access to a terminal that allows scripts to be executed and perform a range of shell scripting functions. Here are some of the noteworthy features of CyberPanel supporting shell scripting:
- Cron Jobs: Schedule your scripts with CyberPanel’s cron job manager.
- Integrated Terminal: Shell scripts can be run directly on the server using CyberPanel’s web terminal, no SSH access required.
- Shell Scripts for Backup and Restore: Use shell scripts to automate backup and restoration of both files and databases in CyberPanel.
With CyberPanel, you can do system admin work like shell scripts with better simplicity, which caters to developers or web or system administrators.
FAQs: Comment in Shell Script
How to add comment in shell script?
You have to use ‘#’ symbol + some text of that comment. Example: # This is a comment.
How to use multi-line comments in shell script?
Multi-line comments aren’t natively supported in shell scripting. That’s for ‘line’ comments, on each line you can use #, and also for block comments, you can use the : ‘… ‘ method.
What is the best place to add comment in shell script?
When writing a script, always have comments at the start of captions what the code does, and also have comments before the complex code sections in order to describe the logic behind it.
Final Thoughts!
Regular commenting in the code is one of the skills every coder should have. Whether you write small utilities or complex automation scripts, comments will help you understand your code better, especially if you come back to it after a gap. Using the best practices and techniques available, you can make sure that your scripts can always be read, well-maintained, and expressed in the most clear way possible.
Start scripting smarter—use comments and streamline your code. Try CyberPanel today!