Security issues should always come first when a web application is developed. Laravel is one of the most popular PHP frameworks, and it comes with built-in features to help developers secure applications. But in terms of security, how secure is Laravel? And what things can you consider for further securing it?
Out of the box, Laravel is a great foundation for security in web applications, but like all tools, it needs to be secured with certain measures. Developers have always had this question: “How do I secure my Laravel website from hackers?” The best thing about this is that, through the several built-in features for security like Content Security Policy (CSP), password hashing, and SQL injection protection, it drastically reduces security breaches. However, for web application protection in a Laravel environment, one needs to ensure proactive measures are taken.
This article will look up securing a Laravel application with the proper tools and techniques. It will discuss security practices you need to set up, like configuring CSP in Laravel, and delve into measures used against SQL injection, cross-site scripting (XSS), and Cross-Site Request Forgery (CSRF). By the end of this article, you’ll know how to keep your Laravel applications secure and minimize their attack surface.
How Secure is Laravel: Laravel Security Features
Laravel has inbuilt features to prevent a lot of common vulnerabilities, standing on its angle for security design. However, as with every framework, no framework can be considered completely secure unless the developers establish the necessary precautions.
- CSRF Protection: With every user session, a unique CSRF token is generated by Laravel for each activity. You cannot use unauthorized submission of forms on behalf of users.
- Password Hashing: By default, passwords are hashed with the bcrypt algorithm, so even if attackers have attained data in the database, no one can read passwords.
- XSS Protection: When views are rendered, all outputs are automatically escaped, which prevents the injection of bad scripts into your pages.
- Encryption: Simple and effective encryption measures are provided by Laravel to ensure that sensitive data present in your applications are secured.
These features create a very promising security platform, but security is more than applying the standard defaults. Let’s see how we can further secure your web application.
Enabling Content-Security-Policy Laravel
One of the best methods to secure your Laravel application from Cross-Site Scripting (XSS) and other script-based attacks is through the use of a Content Security Policy (CSP). CSP confines the sources, from which a webpage can load resources (e.g. javascript, images, and styles), thereby preventing the execution of a malicious script.
Get exclusive access to all things tech-savvy, and be the first to receive
the latest updates directly in your inbox.
Laravel doesn’t implement CSP by default, but it can be easily configured through middleware that handles policy headers.
To implement CSP in Laravel, add headers through middleware. Here’s how you’ll do that:
Create a new CSP Middleware.
First, create a new middleware for handling all
php artisan make:middleware ContentSecurityPolicy
Modify the Middleware
Open the newly created middleware file app/Http/Middleware/ContentSecurityPolicy.php and add the following code to set the Content Security Policy headers:
namespace App\Http\Middleware;
use Closure;
class ContentSecurityPolicy
{
public function handle($request, Closure $next)
{
$response = $next($request);
// Define your Content Security Policy (CSP) rules
$csp = "default-src 'self'; img-src 'self' data:; script-src 'self' https://trusted-source.com; style-src 'self' 'unsafe-inline';";
// Set the CSP header
$response->headers->set('Content-Security-Policy', $csp);
return $response;
}
}
In the example above, we specify that scripts can be loaded only from the same origin (‘self’) or from https://trusted-source.com. Styles may only be loaded from the same origin, but inline styles are allowed with ‘unsafe-inline’. This is a starting point, but you should customize your CSP policy to fit your specific needs.
Register the Middleware
To activate this middleware, register it in the app/Http/Kernel.php file. Add the following line to the web middleware group:
'web' => [
\App\Http\Middleware\ContentSecurityPolicy::class,
// other middleware...
],
Test Your CSP Implementation
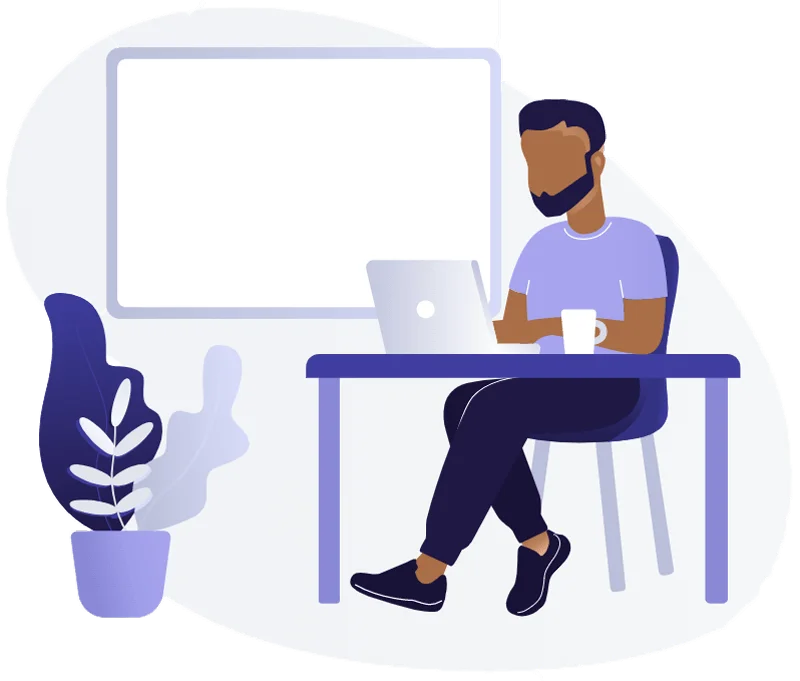
Upon adding the middleware, you’re ready to test your application with the use of built-in developer tools in the browser. Pull up Google Chrome and look under the console ‘Network’ tab to see the CSP header under which your requests are running. Also, use the application to test proper resource loading while blocking any malicious script injection attempts.
How To Secure Laravel Website from Hackers
Here are a few such basic steps to protect your Laravel application:
Keep Your Laravel and Dependencies Up to Date:
Keeping the framework updated makes sure that any patch-related vulnerabilities are fixed. Basically, Laravel opens frequent updates for security fixes. Update all your dependencies by managing them with Composer:
composer update
Besides this, periodically check any known vulnerabilities for the packages you are using by visiting sites like Packagist or SensioLabs Security Checker.
Enable HTTPS:
Always communicate data transmitted over the user’s browser and your server using an HTTPS (SSL/TLS) connection. You can compel HTTPS within Laravel by changing the AppServiceProvider:
public function boot()
{
if (config('app.env') === 'production') {
\URL::forceScheme('https');
}
}
After the installation, you can use two-factor authentication via packages like Google2FA.
Backup and Encryption:
Regular database backups and strong encryption for sensitive data are basic prerequisites that should be satisfied during a compromise. Either use Laravel’s built-in database encryption or tools like Laravel’s EncryptCookies middleware to keep sensitive data encrypted.
Limit Sensitive Routes:
Sensitive routes should be limited to access only to users authorized to those sensitive areas. Use the authentication and authorization means provided by Laravel to restrict access to the crucial parts of your application.
Implement two-factor authentication (2FA):
public function boot()
{
if (config('app.env') === 'production') {
\URL::forceScheme('https');
}
}
However, an extra layer of security is making it difficult with the two-factor authentication. You can use Laravel/UI for setting up 2FA for your users using the built-in package of Laravel:
Database Backup and Encryption
Regular database backups and appropriate encryption for sensitive data against breach entry are very critical. For instance, using Laravel’s built-in database encryption features, or other tools that add extra security, such as Laravel’s EncryptCookies middleware, to keep sensitive pieces of data encrypted.
Is Laravel Secure
Yes, Laravel is a framework that generally has security. However, everyone should keep one thing in mind: security heavily relies on the way it is implemented. However, compliance with the built-in security features and best practices could also make Laravel highly secure.
Takeaways:
- By default, Laravel protects from SQL injections, Cand SRF attacks, and ensures defenses against XSS code.
- Your application will be secured even further by enabling things like CSP and SSL/TLS.
- Updates and strong authentication mechanisms, like two-factor authentication, keep unauthorized users from intruding on resources.
- In summary, Laravel does give a good base, but it is still your responsibility to secure your website. Following the best practices and current trends in security will ensure that bad people will not compromise your Laravel application.
Laravel Security Packages
Aside from offering default security features, Laravel provides a range of security packages for further strengthening your application’s security.
- Laravel Security Headers: This package simplifies the inclusion of security headers such as Content-Security-Policy, Strict-Transport-Security, and many more within your application.
- Laravel Firewall: Configure protection for your app with an in-built firewall that denies access to malicious IPs or limits the use of certain routes.
- Laravel 2FA: This package makes it simple to add an extra step of security to your authentication system through Two-Factor Authentication (2FA).
Laravel Authentication System
The nifty selling point of the authentication system in Laravel is how easy to implement and customize it to fit the needs of applications. The built-in features of the Laravel Authentication System answer login and registration, as well as any password reset functionality you may need, and they are outlined with security.
Bcrypt is employed by Laravel for the hashing of passwords to ensure that even if a hacker breaks into the database, they cannot retrieve the actual passwords. Here’s a rough idea of how an application should be done with Laravel for authentication purposes:
Example: Laravel Authentication
use Illuminate\Support\Facades\Hash;
public function register(Request $request)
{
$request->validate([
'email' => 'required|email|unique:users',
'password' => 'required|min:8|confirmed',
]);
// Hash the password before storing it
$user = User::create([
'email' => $request->email,
'password' => Hash::make($request->password), // Hashed password
]);
auth()->login($user);
return redirect()->route('dashboard');
}
In registering users, their passwords are hashed and just dumped in the database. When a hacker steals the database, they only get the hashed password and it’s useless to them.
Mitigating Laravel Security Vulnerabilities from CSRF
Cross-Site Request Forgery (CSRF) can be defined as a process of tricking the victim into submitting the request forms unknowingly by a malicious user. This form of attack is blocked by built-in measures provided by Laravel, of course, but developers must also ensure that these measures are correctly implemented and activated.
Turning on CSRF Protection in Laravel
CSRF protection is integrated into Laravel by default. Submitted forms are checked by Laravel for the token, whether it is valid or not.
<form method="POST" action="{{ route('updateProfile') }}">
@csrf
<!-- Form fields here -->
<button type="submit">Submit</button>
</form>
The @csrf directive creates hidden input fields along with a token of CSRF. Upon submission of the form, Laravel tests if the token matches the token in the session. If it doesn’t, the request is rejected.
Protection Against XSS (Cross-Site Scripting)
Cross-Site Scripting is an attack that injects malicious scripts into the web pages that are viewed by the other users. Larvel is here to mitigate that issue.
Example: Output Escaping
{{-- Laravel automatically escapes content to prevent XSS --}}
<h1>{{ $user->name }}</h1>
If malicious javascript is been given as a name to a user, Laravel will make it escape the output such that the script will not run. For example, if the name is <script>alert(‘hacked’);</script>, it will not be executed but will just output it as plain text.
SQL Injection Prevention in Laravel
SQL Injection occurs when a hacker is able to manipulate SQL queries by embedding a piece of malicious code in input fields. Prepared statements and Eloquent ORM are the ways of preventing injections in SQL by default in Laravel. Even while using Eloquent or Laravel’s query builder, the framework escapes the user input for the safety of the SQL queries.
For example: Safe Query with Eloquent
// Eloquent query: Laravel automatically escapes inputs
$user = User::where('email', $request->email)->first();
In the above example, Laravel inherently uses prepared statements for this query, and therefore it is safe from SQL injection.
Example: Safe Query with Query Builder
// Query Builder: Laravel escapes input for SQL safety
$user = DB::table('users')->where('email', $request->email)->first();
In the above examples, Laravel ensures that the user email is safely incorporated in the SQL query and prevents the query from being manipulated by the attackers or being executed through arbitrary commands.
Tool to Identify Vulnerabilities: Laravel Security Checker Tool
Thus, one needs to evaluate the potential vulnerabilities an attacker might exploit from your Laravel application from time to time. Laravel security checkers come in handy for developers who want to analyze the condition of their Laravel application in regard to security, where it checks for outdated dependencies and security vulnerabilities.
What is Laravel Security Checker?
Laravel Security Checker is a tool that is able to find security vulnerabilities in the dependencies of a Laravel project. Using it allows for better performance and improved security of your project. Whenever a project uses an outdated version of any package, this tool will check for it and provide the option of upgrading it to a more sophisticated version.
Install the Package: First, you have to install the package via Composer to start using the security checker.
composer require --dev robertovillela/laravel-security-checker
Run the Checker: After installing the component, you can run the security checker with the command given below:
php artisan security:check
This command checks your project dependencies against a list of known vulnerabilities within public repositories.
Report Assessment: The output will list any vulnerable dependencies and all recommended actions. You will also find the issues related to the affected package and a suggestion for updating to a server version.
Update the Packages: If any vulnerabilities are found, update your packages as per the recommendation. Do this by running:
composer update
If this vulnerability is on a Laravel package, check for a patch or new version on either Packagist or GitHub.
Advantages of a Security Checker
- Discovers Vulnerability: It can quickly be discovered while fixing security holes in dependencies.
- Saves Time: Automates known vulnerability checking.
- Great Security Improvement: Keeps your Laravel application updated to the most secure version of libraries.
What’s New in Laravel 11 Security?
Laravel 11 came out with lots of new features in the framework as well as a host of new enhancements. Laravel 11 does not only concern itself with being productive for developers but also guarantees the security of the applications built using this great framework. Here’s what’s new in Laravel 11 security:
Key Security Aspects in Laravel 11
- Password Auto Encryption: Laravel 11 further adds that all passwords are by default set to use bcrypt hashing algorithm making it even tougher for attackers to decrypt passwords even with access to the database.
- CSRF Protection Improved: Laravel 11 further improved the CSRF token handling, thereby reducing the chances of token reuse or tampering. It performs token validation in a more secure manner and better protects sessions.
- Rate Limit Improvements: More granular control of the rate limit is offered in Laravel 11. You can limit the API requests per user or IP address to prevent brute force attacks against it.
- Better Security Headers: Important HTTP Security Headers, such as Strict-Transport-Security (HSTS), Content-Security-Policy (CSP), and X-Content-Type-Options headers, are set automatically in Laravel 11, mitigating XSS and other attacks.
- Enhanced Encryption Features: So awesome, new encryption features added to the latest version of Laravel include being able to use AES-256 encryption because of even more security options.
This means that developers will be able to implement these Features to the full measure of security significance found in the new Laravel version, hence enhancing the web application to a burglar-proof standard.
Laravel Security Best Practices: Create a Powerful and Secure App
Platform security does not end with built-in features; rather, security enhancements in Laravel adopt best practices to prevent common vulnerabilities and save user data. Here are some of the best practices with regard to Laravel security that a developer must follow.
1. Store Sensitive Data Using Environment Variables
- Why it is important: By writing credentials and other secrets related to the database along with API keys directly in cthe ode base, one puts his application in danger from different kinds of security attacks.
- Best practices: Store all confidential information in .env files and access using the Laravel config() helper.
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=your_database
DB_USERNAME=your_username
DB_PASSWORD=your_password
2. Validate All Inputs Entered by Users
- Why it is important: Proper validation ensures that the input is safe and in the right format, which can prevent XSS and SQL Injection attacks.
- Best practice: Validate user input always through Laravel’s built-in validation mechanism.
$request->validate([
'name' => 'required|string|max:255',
'email' => 'required|email|unique:users',
]);
3. Use HTTPS in Production
- Importance: With HTTPS, the data exchanged between the client and server will be encrypted and safeguard the facility of man-in-the-middle attacks.
- Best practice: In AppServiceProvider, use Laravel’s forceScheme method to switch production to HTTPS.
public function boot()
{
if (config('app.env') === 'production') {
\URL::forceScheme('https');
}
}
4. Take Advantage of CSRF Protection Built Into Laravel
- Importance: CSRF tokens prevent unauthorized requests on behalf of authenticated users by an attacker.
- Best practice: Use the @csrf directive in forms, which automatically adds CSRF tokens.
<form method="POST" action="{{ route('submit') }}">
@csrf
<button type="submit">Submit</button>
</form>
5. Keep Dependencies Up-To-Date
- Why it is so important: In an outdated state, these dependencies contain vulnerabilities that can easily be exploited by an attacker.
- Best Practice: Run Composer update to update your Composer dependencies regularly and keep abreast of security patches.
6. Use Strong Passwords
- Reason: Access through weak passwords can easily be cracked.
- Best Practice: Make use of Laravel’s embedded bcrypt hash and consider implementing Two-Factor Authentication (2FA) to enhance security.
Laravel Security Cheat Sheet: Quick Reference
The Laravel Security Cheat Sheet helps developers quickly find the essential resources needed to secure their Laravel applications. Below is a summary of the most critical security practices, methods, and hints to consider while creating a strong application.
Download the PDF of the Laravel Security Cheat Sheet here.
Key Points from the Cheat Sheet:
- Clear User Input: For linear clearing of user input data, use Laravel Validation.
- Enable CSRF Protection: @csrf directive in forms.
- Always Use HTTPS: Enforce the use of HTTPS in production.
- Escape Output: Output user-generated content safely using {{ }} syntax.
- Use Prepared Statements: Seek raw SQL queries to avoid SQL Injection.
- Update Dependencies Consistently: Update Dependencies with composer update as it’s required.
- Set Secure Headers: Add Security Headers Such As X-Content-Type-Options and Strict-Transport-Security (HSTS).
These cheat sheets will provide a quick insight into security mandates and best practices toward expected compliance with security standards in your Laravel applications.
For more details, explore the OWASP Cheat Sheet Series
What CyberPanel Does in Laravel Security
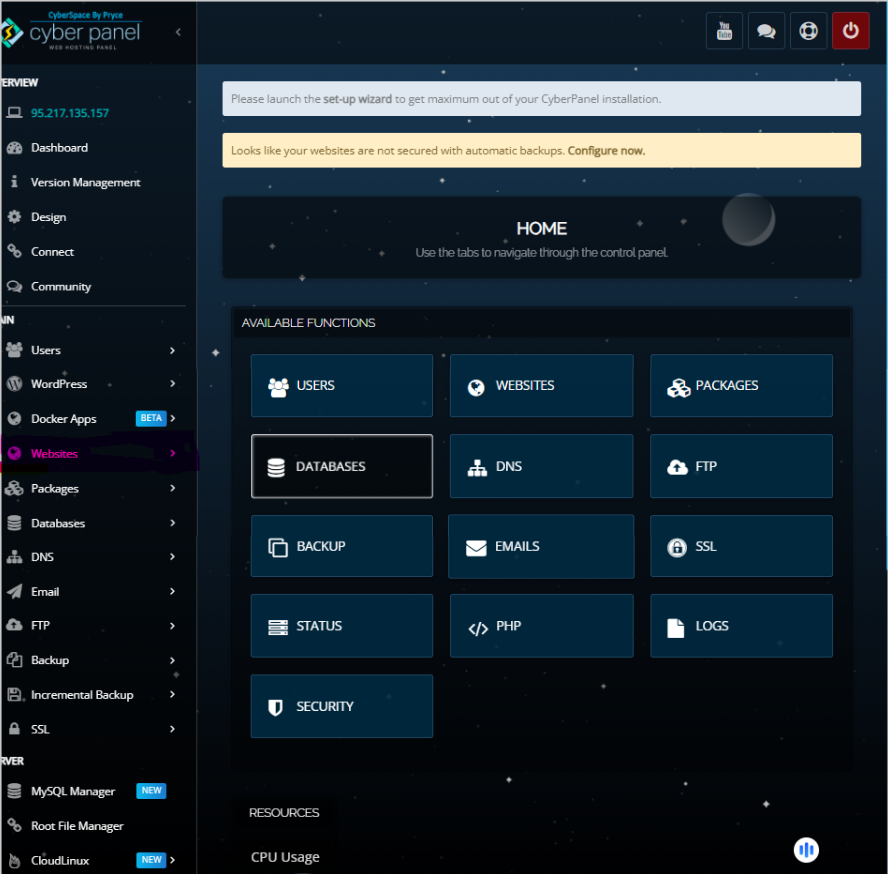
CyberPanel is a web hosting control panel that makes high-speed applications. But more importantly, CyberPanel significantly adds up to the security of applications built on Laravel. It gives many features without compromising Laravel’s built-in security measures and at the same time ensures a very secure host environment. Here are the core roles of CyberPanel:
- SSL/TLS Encryption: CyberPanel would thus make it very much easier to install an SSL certificate for Laravel applications to create security when connections are established over HTTPS.
- Firewall: Protects your server by blocking malicious IPs, preventing brute force and DDoS attacks.
- Two-Factor Authentication (2FA): Adds an extra layer of security to the CyberPanel interface, making sure that only authorized end-users can manage Laravel applications.
- Backup and Restore: Scheduling an automatic backup will create backup copies regularly and give you instant access to restoring your Laravel applications to a secure state after their attacks.
- PHP Security Configuration: You can configure settings around PHP in CyberPanel to disable unsafe functions, limit files uploaded to the host server, and boost the security of the application.
Once all of these open up, then the real magic happens: this is by integrating CyberPanel’s security tools in conjunction with Laravel’s internal protections; you can create a very secure atmosphere for your applications.
FAQs: Laravel Security
1. How to safeguard an application against SQL injection via Laravel?
The use of Eloquent ORM and Query Builder enables Laravel inbuilt escaping of user inputs, which renders SQL injection attempts nearly impossible. It is by not using the raw queries that one can stay invisible from SQL injection attacks.
2. What does CSRF stand for, and how does the framework understand it in Laravel?
Cross Site Request Forgery (CSRF) constitutes an attack that a malicious user tricks a victim to perform on behalf of the attacker. Laravel uses an automatic generation of CSRF tokens to submit forms and verifies these tokens to ensure that the request is legitimate.
3. How does Laravel protect your application from XSS?
Any kind of output to the views decorated with {{ }} gets automatically escaped, thereby protecting a user from cross-site scripting by ensuring that no scripts are injected into a user’s web browser from web pages.
4. What are the best security practices a Laravel application must follow?
The recommended practices for securing a Laravel application include the following:
Keep the Laravel platform and its dependencies up to date.
Use HTTPS communication so that everything is encrypted.
Activate Two-Factor Authentication (2FA) on all admin accounts.
Validate and delete using filters for all user inputs.
Backing up will surely be needed once in a while. It is important to set correct permissions for file and directory locations.
Wrapping Up!
Secure Your Laravel Application: Get Best Practices for Strong Protection
Securing your Laravel application will preserve the essential part of the integrity of your web environment. Using the built-in features of Laravel, as well as the use of CyberPanel, will develop a very robust, strong application that is not compromised or employed toward almost every type of common attack like SQL Injection, CSRF, and XSS.
These are just some of the ways to genuinely enhance the already robust security of a Laravel application: SSL/TLS encryption, time-to-time updates to the dependencies, Two-Factor Authentication (2FA), and firewall settings. It is all set to make your application secure.
Take a step now! These practices set the security of your Laravel application and keep the web environment protected from emerging threats. Start today and secure your Laravel project for the future!
Need help? You can live chat with us now for personalized advice and support to secure your Laravel application and optimize your web hosting environment! Let’s talk and protect your project together.