Do you want to fuse React’s dynamic applications with the strong content management capabilities that WordPress offers? You want to build a website that uses the mega and hyper-modern interactive interfaces of React and the powerful backend of WordPress. If you are a developer after flexibility or a good site for a business owner, this combined expertise of React and WordPress may provide the best innovation yet.
In this guide, you will learn how to use React JS with WordPress to create customized plugins and themes and understand why this combination is becoming so important in building websites. Whether you are considering building a React front-end or a WordPress plugin powered by React, we have it all figured out for you. Let’s take that journey into sharpening our web development skills in WordPress and React.
Why Combine React with WordPress?
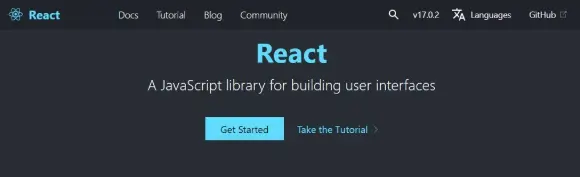
WordPress has a simple interface and extends beyond that with thousands of available plug-ins, while React is very good at the creation of user interfaces that are dynamic, fast, and interactive. The combination gives you:
- Interactive and Dynamic User Interfaces: React is perfect to build for the kind of UIs that need to be highly interactive and dynamic. Combine it with WordPress, and the developer can re-live the experience of an engaging site where the front end appears to change without page reload. A real benefit is this in e-commerce stores or social media platforms where items are constantly refreshing.
- Reusable Components: Create a unique component that can be used anywhere meaning saves you time and consistency.
- Performance Boost: React’s virtual DOM ensures that rendering is faster and improves performance on the website by taking the extra load off WordPress’s backend.
- Headless CMS Flexibility: WordPress as a headless CMS keeps the Content Management layer freely separating the presentational layer so that developers can freely adopt React in developing dynamic front-ends.
- Future-Proof Development: React is assured to keep the website modern and relevant for future times change as it is backed by Facebook and a massive community.
- Improved SEO Capabilities: Frameworks such as Next.js or Gatsby can facilitate the SEO optimization of React websites by absorbing the WordPress tools.
- Vast Ecosystem and Community Support: Both platforms offer massive documentation and vast community support services to solve problems and improve development.
- Mobile Development as well: There is Cordova that helps leverage this transition using React Native with React to move your WordPress site smoothly into a mobile application.
- While Cordova can serve as a quick wrapper, production-grade apps usually benefit from a richer component set, native navigation, and tighter performance budgets. That’s where React Native shines: it lets you reuse much of your WordPress-powered React logic while still delivering truly native UI elements on iOS and Android.
- If your team lacks in-house mobile expertise, you can hire dedicated developer on react native to bridge the gap. A specialized React Native engineer will turn your existing REST (or GraphQL) endpoints into fast, touch-friendly views, implement offline caching for posts and WooCommerce products, and integrate push notifications without disrupting your core web roadmap.
- The result is a single content pipeline powering both the desktop web and the mobile app, shorter time-to-market, and a consistent brand experience across all channels.
Setting Up React JS With WordPress
Prerequisites
- Installation of the software presently needs to be completed, such as
- Your Tools Installed
- A code editor (like VS Code) WordPress (local/server installed) and Node.js and npm
Step 1: Install and Activate WordPress REST API
You can use a built-in REST API to fetch data from WordPress. Ensure the WordPress site has enabled REST API access as follows:
- Log in to the WordPress admin panel.
- Settings > Permalinks, set to post name.
Step 2: Create a React App
Create-react-app is an easy way to bootstrap a React app:
Get exclusive access to all things tech-savvy, and be the first to receive
the latest updates directly in your inbox.
npx create-react-app react-wordpress-app
cd react-wordpress-app
Step 3: Fetch WordPress Data Using REST API
Install axios for consuming the HTTP requests:
npm install axios
Here’s an example you could use to fetch posts from your WordPress site:
import React, { useState, useEffect } from 'react';
import axios from 'axios';
const App = () => {
const [posts, setPosts] = useState([]);
useEffect(() => {
axios.get('https://yourwordpresssite.com/wp-json/wp/v2/posts')
.then(response => setPosts(response.data))
.catch(error => console.error('Error fetching data:', error));
}, []);
return (
<div>
<h1>WordPress Posts</h1>
{posts.map(post => (
<div key={post.id}>
<h2>{post.title.rendered}</h2>
<p dangerouslySetInnerHTML={{ __html: post.excerpt.rendered }} />
</div>
))}
</div>
);
};
export default App;
Output
The React app would now dynamically show the titles and snippets of WordPress posts.
A Quick Guide to Build WordPress Plugin With React
Developing a plugin with React opens up the avenue of including React functionalities in your WordPress site. Follow these steps:
Step 1: Create Your Plugin Folder
- Move to wp-content/plugins/.
- Create a directory named something like react-plugin.
- Inside that folder, create a file called: react-plugin.php:
<?php
/**
* Plugin Name: React Plugin
* Description: A simple plugin using React.
* Version: 1.0
* Author: Your Name
*/
function load_react_script() {
wp_enqueue_script(
'react-plugin-script',
plugin_dir_url(__FILE__) . 'build/index.js',
['wp-element'], // Ensure React is loaded.
null,
true
);
}
add_action('wp_enqueue_scripts', 'load_react_script');
Step 2: Create the React App
While this is possible through the command line, it can be done right from the plugin folder:
- Create the React app:
npx create-react-app react-plugin
cd react-plugin
- Now, just move that build folder to your plugin directory.
npm run build
Step 3: Activate the Plugin
Now activate the plugin from your WordPress admin panel and your React app runs as part of your WordPress installation.
Developing a WordPress Theme with React
You can build WordPress theme with React without installing any bulky plugins and give it an awesome wiggle into the design and functionality.
Step 1 – Create a Theme Folder
- Step inside the wp-content/themes/ directory
- Create a folder, e.g. react-theme.
- Add a style.css file:
/*
Theme Name: React Theme
Author: Your Name
Description: A WordPress theme powered by React.
Version: 1.0
*/
Step 2 – Create a Basic PHP File
Create an index.php file:
<?php
echo '<div id="root"></div>';
wp_enqueue_script(
'react-theme-script',
get_template_directory_uri() . '/build/index.js',
['wp-element'],
null,
true
);
?>
Step 3 – Introducing React
- Create a React application under your theme folder:
npx create-react-app react-theme
cd react-theme
npm run build
- Copy the build folder into your theme directory.
- Activate it via the admin panel in WordPress.
Advanced Integrative Techniques to Explore
For advanced integration between React and WordPress, try the next:
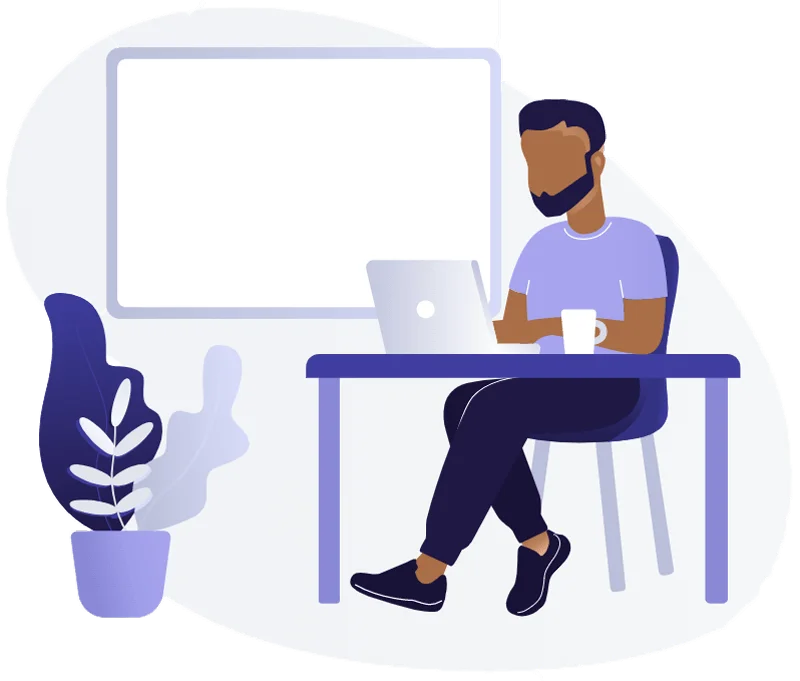
Fetching using WP REST API with React
The WordPress REST API exposes WordPress content to outside world for use. By fetching contents from the REST API, you could make a React front-end that dynamically displays WordPress contents.
Demo: Fetch Posts with React
import React, { useState, useEffect } from 'react';
import axios from 'axios';
const Posts = () => {
const [posts, setPosts] = useState([]);
useEffect(() => {
axios.get('https://yourwordpresssite.com/wp-json/wp/v2/posts')
.then(response => setPosts(response.data))
.catch(error => console.error('Error fetching posts:', error));
}, []);
return (
<div>
<h1>Blog Posts</h1>
{posts.map(post => (
<div key={post.id}>
<h2>{post.title.rendered}</h2>
<div dangerouslySetInnerHTML={{ __html: post.content.rendered }} />
</div>
))}
</div>
);
};
export default Posts;
Custom Post Types by React
Custom Post Type (CPT) extends the WordPress default functionality and enables you to use various contents like portfolios, testimonials, or products. In React you dynamically render these custom content types.
Demo: Registering a Custom Post Type in WordPress
Drivelling these lines in functions.php of your theme:
function create_custom_post_type() {
register_post_type('portfolio',
array(
'labels' => array(
'name' => __('Portfolios'),
'singular_name' => __('Portfolio'),
),
'public' => true,
'has_archive' => true,
'show_in_rest' => true, // Enable REST API support
)
);
}
add_action('init', 'create_custom_post_type');
Using the REST API fetch these and show them
Custom Post Types in React
import React, { useState, useEffect } from 'react';
import axios from 'axios';
const Portfolio = () => {
const [portfolios, setPortfolios] = useState([]);
useEffect(() => {
axios.get('https://yourwordpresssite.com/wp-json/wp/v2/portfolio')
.then(response => setPortfolios(response.data))
.catch(error => console.error('Error fetching portfolios:', error));
}, []);
return (
<div>
<h1>Portfolio</h1>
{portfolios.map(portfolio => (
<div key={portfolio.id}>
<h2>{portfolio.title.rendered}</h2>
<div dangerouslySetInnerHTML={{ __html: portfolio.content.rendered }} />
</div>
))}
</div>
);
};
export default Portfolio;
Using React to Build Headless WordPress
Headless WordPress is where the front-end (React) is separate from the back-end (WordPress). A real fast modern user experience using WordPress as a content management system.
Stepwise Guide
WordPress as Headless CMS:
Always enable REST API to pull data.
Create a React Front-End:
Develop the front-end by separating it using React.
Deploy Both Immediately from each other:
With a netlify for React platform separately and a traditional hosting for WordPress.
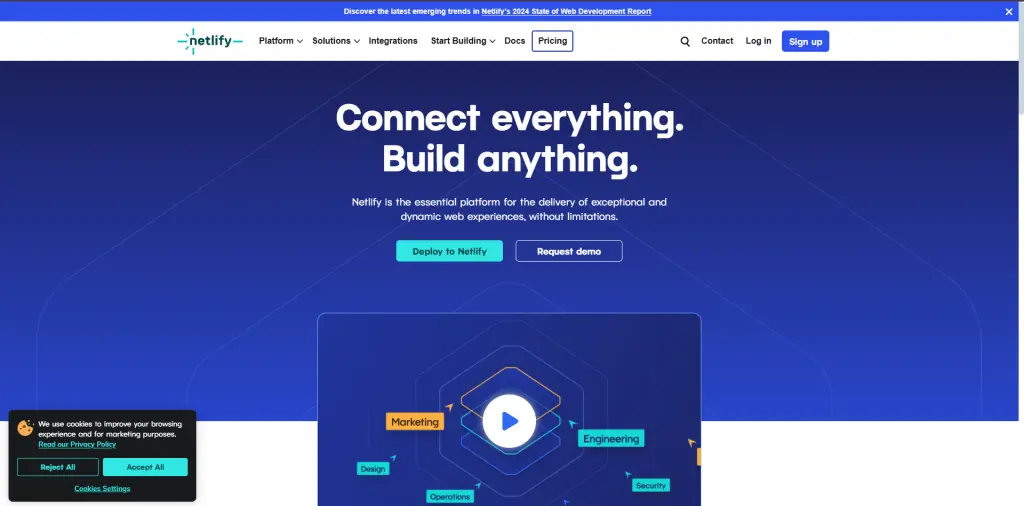
SEO Best Practices for React with WordPress
Well, since React is a single page from its SEO perspective, integrating this site would again work well with that, as dynamic and search-engine friendly.
SEO Tips
- Server-Side Render: Render react components server-side with Next.js.
- Static Site Generation: Pre-rendering pages at build time to achieve speed.
- Managing metadata: Use WordPress plugins for SEO metadata management and bring it to React components.
- Dissemination of Content: Caching and CDNs to make the delivery of content faster.
Using React for WordPress Widgets
WordPress widgets are made available for bringing exciting content sidebars or anywhere else widget-ready.
Example: Create a Widget by React
Register a widget area in WordPress:
function register_widgets() {
register_sidebar(array(
'name' => 'React Widget Area',
'id' => 'react_widget_area',
'before_widget' => '<div>',
'after_widget' => '</div>',
));
}
add_action('widgets_init', 'register_widgets');
Then Render the React Widget in the Registered Area:
function enqueue_react_widget() {
wp_enqueue_script(
'react-widget',
get_template_directory_uri() . '/build/index.js',
['wp-element'],
null,
true
);
}
add_action('wp_enqueue_scripts', 'enqueue_react_widget');
Benefits of Using React with WordPress
1. Interactive and Dynamic User Interfaces
React is, as you may know, a platform for implementing highly interactive and dynamic UIs. Used with WordPress, developers can create a user experience where the front-end does not reload but updates seamlessly within itself, say, an e-commerce app or social media app, within other such real-time updates.
2. Reusable Components
By allowing the construction of reusable UI elements, React saves development time with consistency all over the website. Maintenance becomes simpler and scalability is achieved as your project grows.
3. Performance Upgrade
React’s virtual DOM results in fast updates and rendering, which results in improved performance for the site. So while using WordPress, most of the client interactions would take place on the client side and would reduce the load on WordPress’s backend.
4. Flexibility of a Headless CMS
Using WordPress as a headless CMS means that developers can separate content management (backend) from presentation (frontend) using React. It allows flexibility to use different technologies for both components while enjoying a solid experience in CMSs.
5. Development Future-Proof
React is the most modern and brilliant library of JavaScript, with backing from Facebook and a larger developer community. Its use within WordPress makes your website modern in every development practice. It is thus easier to adapt to future trends.
6. Enhance SEO Capability
When combined with server-side rendering (SSR) or static site generation (SSG) through frameworks such as Next.js, React gives better SEO performance. WordPress is already an SEO tool and when combined with React, it is possible to take high-profile websites to the next level of optimization.
Alternatives to Use Against React with WordPress
1. Vue.js with WordPress
Vue.js is also a popular JavaScript framework known for its simplicity and flexibility. It provides:
- Small Learning Curve: Easier to learn in comparison to React.
- Smooth Integration: Like React, Vue works well with the WordPress REST API.
2. Angular with WordPress
Angular, Google’s backed framework, is a complete solution for building large-scale applications. Its features include:
- Full Framework: Out-of-the-box routing, HTTP services, and more.
- Two-Way Data Binding: This makes it easier for the model and views to be synchronized.
3. Svelte with WordPress
Svelte is a newer framework that compiles components at compile time into optimized javascript. Major benefits include:
- Better Performance: Faster run because there is no virtual DOM involved.
- Simpler Syntax: Much simpler than using React or Vue.
4. Gatsby with WordPress
Gatsby is a static site generator that works with WordPress to build extremely performing sites that are optimized for searching. Benefits include:
- Lightning Speed Load: Pre-rendered static pages.
- Improved SEO: Better for search engine indexing.
5. Native WordPress with jQuery
This isn’t so much modern, but old jQuery-powered WordPress is still capable of meeting some basic dynamic estate requirements. However, the performance and scaling compared to React or those frameworks are poor.
Common Challenges and Their Solutions
1. Limitations of REST API
Powerful, but has impacts such as incomplete data and bottlenecks in performance dependent on the amount of data loaded by the WordPress REST API.
Solutions:
- Custom endpoints for fetching data using register_rest_route extend the REST API.
- Using GraphQL (via plugins like WPGraphQL) for more flexible data queries.
2. SEO Issues of React
If the content is rendered by the client side, an SEO-unfriendly environment is created wherein search engine bots are unable to index content accurately.
Solution:
- The server side may use Next.js or Gatsby for server-side rendering (SSR) or static site generation (SSG).
- Dynamic metadata can also be incorporated with the use of WordPress plugins such as Yoast SEO and React Helmet.
3. Complexity of Integration
It can be quite tricky dealing with integration between React and WordPress, especially for developers who are void of knowledge in either of the platforms.
Solution:
- A solution like Frontity can be used since it is a completely React-based framework designed specifically for WordPress.
- Following the best practices in folder structure and reusability of components.
4. State and Data Management
It is a well-known fact that data synchronization is quite complicated between WordPress and React, especially when the applications are larger.
Solution:
- State management should be done efficiently using Redux or React Context API:
- Data at the API level is fetched and cached to optimize the API calls.
5. Bottlenecks of Performance
A large dataset results in a single rendering on an application or it holds up the React application due to many API calls in a short time.
Solutions:
- The data can be chunked and loaded by implementing pagination or infinite scrolling.
- It can optimize API calls in aggregative queries and keep the responses cached.
6. Security Threats
Headless Content Management Systems like WordPress are prone to some kind of security holes, for example, leaking sensitive information through the REST API.
Solution:
- Restrict API access with authentication mechanisms like OAuth2 or API keys.
- Update WordPress and React dependencies regularly to mitigate known vulnerabilities.
7. Cross-Browser Compatibility
React features can cause inconsistencies among browsers.
Solution:
- Babel polyfills help Browsers that do not support new features.
- Testing of the application on multiple browsers during development.
8. Dependency Management
With WordPress plugins and React libraries, keeping up with what has changed might get tedious.
Solution:
- Automate yours with package managers like npm or Yarn.
- Read changelogs regularly and update your dependencies to the most recent stable versions.
Role of CyberPanel in React with WordPress Integration
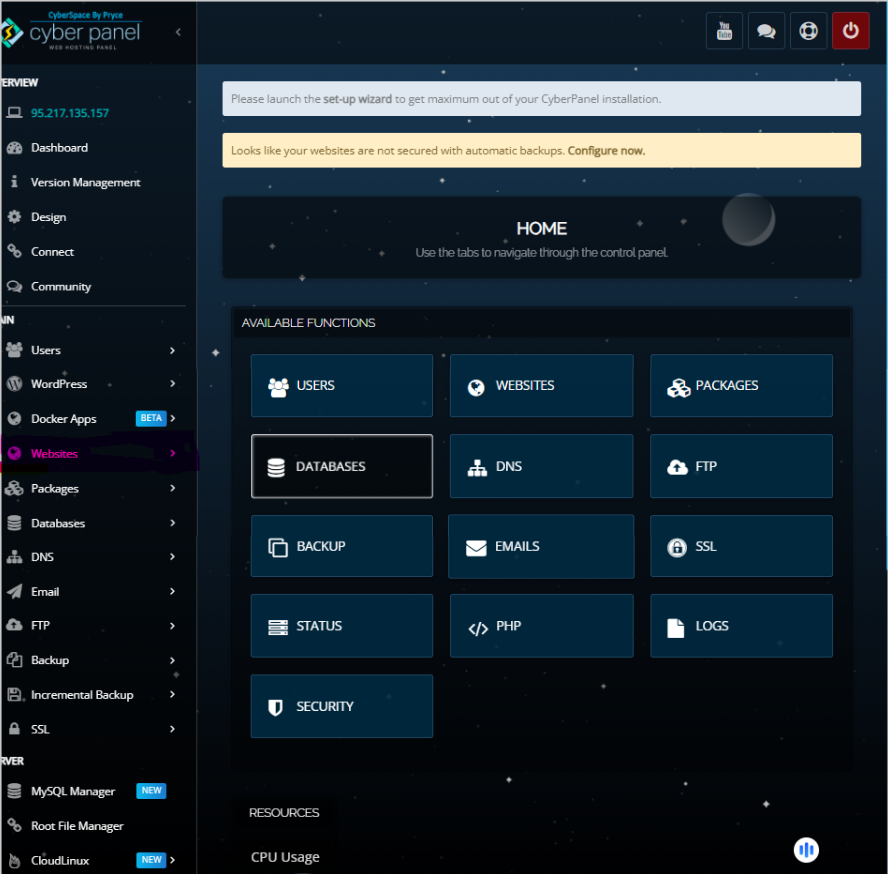
CyberPanel is quite interesting as it offers new-age web hosting control panel facilities to set up and manage various applications in real-time, including WordPress with React. OpenLiteSpeed also powers the installation, ensuring a highly efficient and robust environment that will host dynamic websites. So, this is how CyberPanel contributes to integrating React with WordPress:
1. Performance Optimization
LiteSpeed Cache is designed with CyberPanel to make sure WordPress sites perform excellently. It ensures immediate delivery of content that’s quite a crucial requirement by way of React involving dynamic front-end performance.
2. Smooth SSL Integration
For CyberPanel, there is no hassle in getting or setting up SSL certificates so as to secure the connection between the React front-end and WordPress back-end.
3. Git Deployment
It has great built-in Git support in CyberPanel so app developers can easily build their React applications and facilitate modern development approaches such as version control to backtrack changes, too easily update or rollback whenever necessary.
4. Database Management
Using CyberPanel, one of the more intuitive interfaces is made available for database management to configure WordPress databases with React easier in terms of performance and maintenance.
5. Developer-Friendly Tools
The developer-friendly features of SSH access, PHP management, and staging environments position CyberPanel as the best solution-oriented developer option for projects combining React JS and WordPress.
FAQs for Using React with WordPress
Q1: Would it be possible to use React with an existing WordPress site?
Of course, you could add React functionality to any existing WordPress site. One could use the REST API for fetching data and building interactive components or even go for a headless CMS whereby React would handle the frontend and WordPress manages the backend.
Q2: What is the benefit of WordPress being used by React?
It creates dynamic interfaces, gives React’s “virtual” DOM for fast processing, makes it scalable with architecture, and enables the building of headless CMS setups for full-blown modern web applications.
Q3: Can I create WordPress themes and plugins in React?
Yes, indeed, React can be used for creating themes and plugins in WordPress. Themes would use React dynamic front-end visualization while plugins would empower some custom functionality with the help of React components.
Q4: How will I handle SEO for React with WordPress?
Going for server-side rendering (SSR) frameworks, such as Next.js, will be one way of rendering React to fulfill SEO requirements. Gatsby for static site generation, as well as dynamically managing metadata using React Helmet and WordPress SEO plugins like Yoast SEO would also work.
Q5: What kind of hosting would you advise on React with WordPress?
CyberPanel is certainly among the best hosting platforms that can be used for React and WordPress projects. A complete package when it comes to performance optimization, very easy SSL installation, and developer-friendly tools for modern web applications.
Culmination
Transform the development of the websites using React in WordPress
To wrap up, combining React with WordPress is the next best thing to modern JavaScript and WordPress strength power. It can help you develop scalable, fast, and highly interactive sites for the new digital landscape. It not only covers building custom theming and plugin architectures but also includes the creation of headless content management solutions.
Thus, you can open the gates of harnessing the power of React with WordPress with some other things like CyberPanel for optimized hosting or Next.js for SEO benefits. Whether you’re a developing developer or a Shining business owner, it ends up providing the phenomenal performance and flexibility that makes you stand out.
So, are you ready to level up your website? Explore today the powers of React with WordPress and create the web magic that your users will love!