Docker has completely changed software deployment by offering a lightweight, portable, and consistent runtime environment. For Java developers, Docker offers a seamless way to package and run Java applications across different systems without worrying about compatibility issues.
By using a Docker container for Java applications, you can ensure consistency across different environments, such as development, testing, and production. It also helps simplify dependency management by building Java, libraries, and configurations in a single image. Lastly, it helps improve scalability by running Java applications as lightweight, isolated containers.
In this guide, we will walk through the basics of setting up and running Java applications in Docker, from writing a simple Dockerfile to optimizing the container for performance.
Prerequisites for Running Java in Docker
Before you start containerizing your Java applications, make sure that your system is ready with the following prerequisites:
- Install Docker
Docker must be fully installed on your system to build and run containers. You can install it using the following commands:
- Windows/macOS: Download Docker Desktop
- Linux: Install Docker using your package manager:
sudo apt install docker.io # Debian/Ubuntu
Get exclusive access to all things tech-savvy, and be the first to receive
the latest updates directly in your inbox.
sudo dnf install docker # Fedora
Verify the installation by running, using:
docker –version
- Install Java Development Kit (JDK) (Optional)
While Java is included in the container, having a JDK on your local machine helps in development and testing phases. You can install OpenJDK using:
sudo apt install openjdk-17-jdk # Ubuntu/Debian
sudo dnf install java-17-openjdk # Fedora
Check the installed version with:
java -version
- Basic Knowledge of Docker Commands
Undering basic Docker commands will help you manage containers effectively. Familiarize yourself with these:
- docker build – Build a Docker image
- docker run – Run a container
- docker ps – List running containers
- docker stop – Stop a running container
- docker logs – View logs of a container
- A Simple Java Application
A simple Java application is needed to start containerization. You can run a hello world code as well:
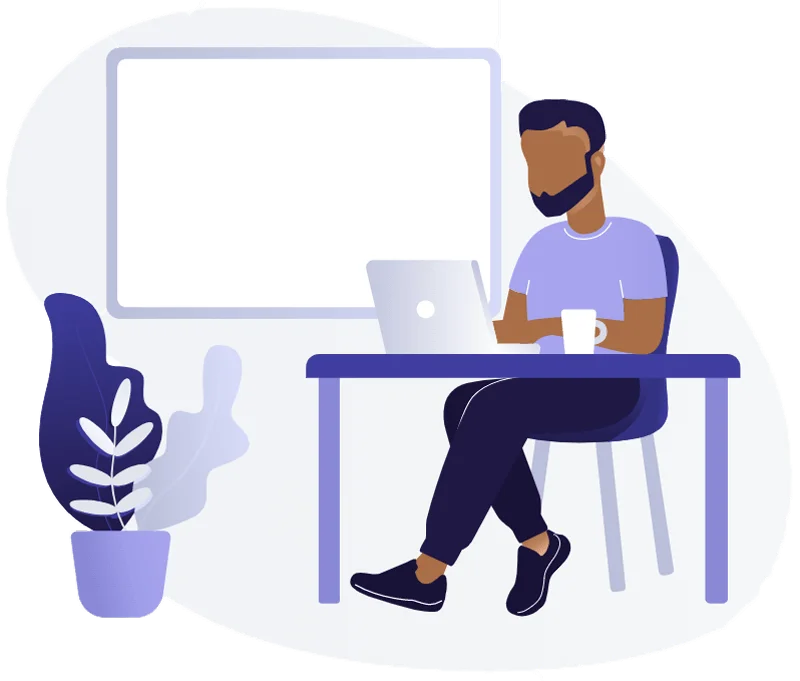
public class HelloDocker {
public static void main(String[] args) {
System.out.println(“Hello from Dockerized Java!”);
}
}
- Dockerfile for Java
A valid Dockerfile is required to define how the Java application runs inside a container. Here is an example:
FROM openjdk:17
COPY HelloDocker.java /app/
WORKDIR /app
RUN javac HelloDocker.java
CMD [“java”, “HelloDocker”]
Setting Up Docker Container for Java Applications
To set up Docker container for Java, you need to cover these essential setup steps:
- Install and Configure Docker
Ensure that Docker is installed and running properly on your system:
- Windows/macOS: Install Docker Desktop
- Linux: Install Docker using the package manager:
sudo apt install docker.io # Debian/Ubuntu
sudo dnf install docker # Fedora
Verify the installation by running:
docker –version
- Enable Non-Root Access (Linux Only)
By default, Docker needs root privileges, to run as non-root follow these steps:
- Add user:
sudo usermod -aG docker $USER
newgrp docker
- Log out and back in to apply the changes.
- Setting Up a Java Project
Create a basic Java project for testing:
mkdir java-docker-app && cd java-docker-app
Inside the directory, create a simple Java file:
// HelloDocker.java
public class HelloDocker {
public static void main(String[] args) {
System.out.println(“Hello from Dockerized Java!”);
}
}
- Writing a Dockerfile for Java
A Dockerfile shows how Docker should build and run your Java applications. Create a new file named Dockerfile in the project directory:
- Use an official OpenJDK base image
FROM openjdk:17
- Set working directory inside the container
WORKDIR /app
- Copy the Java source code to the container
COPY HelloDocker.java /app/
- Compile the Java program
RUN javac HelloDocker.java
- Command to run the Java program
CMD [“java”, “HelloDocker”]
- Build the Docker Image
Run the following command to build your Java application into a Docker image:
docker build -t java-docker-app .
This creates a container image named java-docker-app.
- Run the Java Application in Docker
Now, run the container using:
docker run –rm java-docker-app
If everything is set up correctly, you should see:
Hello from Dockerized Java!
- Managing Java Containers
List running containers:
docker ps
Stop a running container:
docker stop <container_id>
Remove an image:
docker rmi java-docker-app
Building and Running a Java Docker Container
Once you have built a Dockerfile for your Java application, the next steps involve building the Docker image and running the container.
- Building the Docker Image
The Docker image is a screenshot of the Java application that contains everything you need to run it in a container.
Build the image by navigating to the directory where your Dockerfile is saved and run:
docker build -t my-java-app .
This command:
- docker build: Initiates the image-building process.
- -t my-java-app: Assigns the name my-java-app to the image.
- .: Specifies the current directory as the location of the Dockerfile.
Once the build is complete, check if the image is successfully created:
docker images
You should see an entry like:
- Running the Container
After building the image, run an instance by:
docker run –rm my-java-app
- docker run: Starts a new container from the specified image.
- –rm: Automatically removes the container once it stops.
- my-java-app: The name of the Docker image to run.
- Running the Container in Detached Mode
To keep the Docker container for Java running in the background, run the following command:
docker run -d –name java-container my-java-app
Check running containers:
docker ps
To stop the container:
docker stop java-container
- Running a Java Application with Environment Variables
Pass your environment variables when running the container:
docker run –rm -e JAVA_ENV=production my-java-app
Inside your Java application, retrieve it using:
String env = System.getenv(“JAVA_ENV”);
Managing Java Dependencies in Docker
When running Java applications in Docker, it is essential to handle dependencies effectively. You can use a dependency management tool like Maven or Gradle can help streamline this process:
Using Maven in a Dockerfile
FROM maven:3.8.5-openjdk-17 AS builder
WORKDIR /app
COPY pom.xml .
RUN mvn dependency:go-offline
COPY src/ ./src/
RUN mvn package -DskipTests
FROM openjdk:17
WORKDIR /app
COPY –from=builder /app/target/myapp.jar .
CMD [“java”, “-jar”, “myapp.jar”]
Using Gradle in a Dockerfile
FROM gradle:7.3.3-jdk17 AS builder
WORKDIR /app
COPY . .
RUN gradle build –no-daemon
FROM openjdk:17
WORKDIR /app
COPY –from=builder /app/build/libs/myapp.jar .
CMD [“java”, “-jar”, “myapp.jar”]
Optimizing Docker Images for Java Applications
Optimizing Docker image containers helps improve performance and reduces image size:
Key Optimization Techniques
- Use slim base images: Instead of openjdk:17, use openjdk:17-slim.
- Reduce unnecessary layers: Combine RUN commands in the Dockerfile.
- Avoid copying unnecessary files: Use .dockerignore to exclude target/, node_modules/, etc.
- Use multi-stage builds: Keep only the necessary files in the final image.
Using Multi-Stage Builds for Java in Docker
Multi stage builds help create a smaller final image by compiling the application in one stage.
Example: Multi-Stage Build for a Spring Boot App
FROM maven:3.8.5-openjdk-17 AS builder
WORKDIR /app
COPY . .
RUN mvn clean package -DskipTests
FROM openjdk:17-slim
WORKDIR /app
COPY –from=builder /app/target/myapp.jar .
EXPOSE 8080
CMD [“java”, “-jar”, “myapp.jar”]
Exposing and Networking Java Applications in Docker
For web based Java applications, it is crucial to expose ports and networking containers.
Exposing a Java App Port
EXPOSE 8080
Run the container with:
docker run -p 8080:8080 my-java-app
Access the app at http://localhost:8080.
Connecting Multiple Containers with Docker Compose
For Java apps using databases (MySQL, PostgreSQL), use Docker Compose:
version: ‘3.8’
services:
app:
image: my-java-app
ports:
– “8080:8080”
depends_on:
– db
db:
image: mysql:8
environment:
MYSQL_ROOT_PASSWORD: root
MYSQL_DATABASE: mydb
Run with:
docker-compose up -d
Troubleshooting Common Issues
Issue | Cause | Solution |
Container Crashes | Missing dependencies or misconfigured app | Check logs using docker logs my-java-app |
Out of Memory (OOM) | Java heap size too large | Limit memory: -Xmx512m or docker run -m 512m |
Port Conflicts | Port already in use | Use a different port: docker run -p 9090:8080 |
Network Connection Issues | Container not connected to network | Check networks: docker network ls and connect manually |
Slow Startup | Large image size or missing optimizations | Use multi-stage builds and a slim base image |
File Permission Issues | Incorrect user permissions in the container | Set correct ownership with chown and chmod |
Wrapping Up – Docker Container For Java
Creating Docker containers for Java applications with Docker simplifies deployment, enhances scalability, and ensures consistency across different environments. By following this guide, you can easily create and run containers and troubleshoot issues!
Frequently Asked Questions
1. What is the best base image for running Java in Docker?
Popular choices include openjdk
and adoptopenjdk
images, which provide lightweight and optimized Java environments.
2. Can I run multiple Java applications in a single container?
While possible, it’s best to run each application in a separate container following the micro services architecture for better scalability and isolation.
3. Why should I use Docker for Java applications?
Docker provides a consistent environment, simplifies dependency management, and ensures application portability across different systems.